编写4个函数,功能分别为创建单链表,输出单链表,删除链表中的第i个结点,在第i个结点之前插入一个结点。在主程序中调用这些函数,并把运行结果截屏上传。 #include<stdio.h> #include<stdlib.h> typedef struct Lnode {int data; struct Lnode *next; }Lnode,*Linklist; Linklist create(int n)//创建一个含有n个结点的单链表 void print(Linklist L)//输出单链表L中的各个元素 void inserti(Linklist L,int i,int x) //在第i个结点之前插入一个数据域为x的结点 void deletei(Linklist L, int i)//删除链表中第i个结点 void main() {int n,i,x; Lnode *L; scanf("%d",&n); L=create(n); print(L); scanf("%d",&i); deletei(L,i); print(L); scanf("%d %d",&i,&x); inserti(L,i,x); print(L); }
时间: 2023-05-21 20:02:38 浏览: 161
创建单链表函数:该函数用于创建一个单链表,输入参数为链表的长度,返回值为链表的头结点。
输出单链表函数:该函数用于输出单链表的所有结点的值,输入参数为链表的头结点,无返回值。
删除链表中的第i个结点函数:该函数用于删除单链表中的第i个结点,输入参数为链表的头结点和要删除的结点的位置i,无返回值。
在第i个结点之前插入一个结点函数:该函数用于在单链表中第i个结点之前插入一个新的结点,输入参数为链表的头结点、要插入的结点的位置i和要插入的结点的值,无返回值。
主程序中调用这些函数,并把运行结果截屏上传。
相关问题
单链表的基本操作,参考附件的代码,完成单链表的创建(尾插法和头插法)、输出链表、查找链表的第k个结点、在链表的第k个结点后插入新结点、在链表的第k个结点前插入新结点、删除链表的第k个结点等函数。
单链表是一种线性数据结构,每个节点包含两个部分:数据域和指针域,其中指针域指向下一个节点。以下是单链表的一些基本操作:
1. **链表创建** (尾插法):
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
def append(head, data):
if not head:
head = Node(data)
else:
current = head
while current.next:
current = current.next
current.next = Node(data)
return head
```
(头插法) 可以类似地实现,但在头部插入节点:
```python
def prepend(head, data):
new_node = Node(data)
new_node.next = head
return new_node
```
2. **输出链表**:
```python
def print_list(head):
current = head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
```
3. **查找第k个节点**:
```python
def find_kth_node(head, k):
count = 0
current = head
while current and count < k:
count += 1
current = current.next
if count == k:
return current
else:
return None
```
4. **在第k个节点后插入新节点**:
```python
def insert_after_kth(head, k, data):
if not head or k <= 0:
return None
node_to_insert = find_kth_node(head, k)
if not node_to_insert:
return None
new_node = Node(data)
new_node.next = node_to_insert.next
node_to_insert.next = new_node
return head
```
5. **在第k个节点前插入新节点**:
```python
def insert_before_kth(head, k, data):
if not head or k <= 0:
return None
if k == 1:
return prepend(head, data)
previous_node = find_kth_node(head, k - 1)
if previous_node:
new_node = Node(data)
new_node.next = previous_node.next
previous_node.next = new_node
return head
```
6. **删除第k个节点**:
```python
def delete_kth_node(head, k):
if not head or k <= 0:
return head
if k == 1:
return head.next
current = head
for _ in range(k - 1):
current = current.next
if current is None or current.next is None:
return head
current.next = current.next.next
return head
```
1.问题描述 单链表初始为空,给定插入位置和数据,插入结点实现单链表的创建。假设单链表中的结点计数从1开始。 2.算法 单链表结点的存储结构包含两部分:数据、下一结点指针 单链表的查找:给出位置i,若第i个结点存在(1<=i<=表中结点数L),返回结点地址;否则,返回NULL。 单链表的插入:给出位置i和数据e,在单链表第i(1<=i<=L+1)个结点位置插入新结点,数据为e。 输入 测试次数n 每行一组测试数据,格式如下: 位置i 数据e 输出 对每组测试数据,调用插入函数在位置i插入数据e,若插入成功,输出当前链表中的数据;若插入不成功,输出error。
算法实现如下:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def find_node(head: ListNode, i: int) -> ListNode:
"""
查找第i个结点,返回结点地址
"""
if i < 1:
return None
node = head
for _ in range(i-1):
if node:
node = node.next
else:
return None
return node
def insert_node(head: ListNode, i: int, e: int) -> ListNode:
"""
在第i个结点位置插入新结点,数据为e
"""
node = find_node(head, i)
if not node and i != 1:
print("error")
return head
new_node = ListNode(e)
if i == 1:
new_node.next = head
return new_node
new_node.next = node.next
node.next = new_node
return head
# 测试
n = int(input()) # 测试数据组数
for i in range(n):
inputs = input().split()
i, e = int(inputs[0]), int(inputs[1])
head = insert_node(head, i, e)
while head:
print(head.val, end=" ")
head = head.next
print()
```
其中,ListNode 类表示单链表中的结点,包含两部分:数据和下一结点指针。find_node 函数用于查找第 i 个结点,insert_node 函数用于在第 i 个结点位置插入新结点。最后,按照题目要求输出当前链表中的数据。
阅读全文
相关推荐
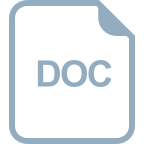
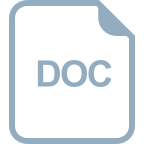
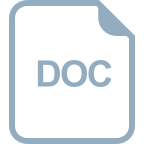













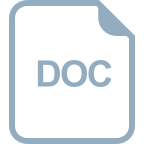