1、 设计一个复数Complex类,用友元函数重载乘法运算符(*),用成员函数重载除法运算符(/),完成两个复数对象的乘除法。
时间: 2024-03-02 11:49:59 浏览: 70
好的,我来解答你的问题。
下面是复数Complex类的定义和实现:
```cpp
#include<iostream>
using namespace std;
class Complex {
private:
double real; // 复数的实部
double imag; // 复数的虚部
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator*(const Complex& c2); // 重载乘法运算符
Complex operator/(const Complex& c2); // 重载除法运算符
void display(); // 显示复数
friend Complex operator+(const Complex& c1, const Complex& c2); // 友元函数重载加法运算符
friend Complex operator-(const Complex& c1, const Complex& c2); // 友元函数重载减法运算符
};
Complex Complex::operator*(const Complex& c2) {
Complex c;
c.real = this->real * c2.real - this->imag * c2.imag;
c.imag = this->real * c2.imag + this->imag * c2.real;
return c;
}
Complex Complex::operator/(const Complex& c2) {
Complex c;
double d = c2.real * c2.real + c2.imag * c2.imag;
c.real = (this->real * c2.real + this->imag * c2.imag) / d;
c.imag = (this->imag * c2.real - this->real * c2.imag) / d;
return c;
}
void Complex::display() {
if (imag < 0) {
cout << real << " - " << -imag << "i" << endl;
} else {
cout << real << " + " << imag << "i" << endl;
}
}
Complex operator+(const Complex& c1, const Complex& c2) {
Complex c;
c.real = c1.real + c2.real;
c.imag = c1.imag + c2.imag;
return c;
}
Complex operator-(const Complex& c1, const Complex& c2) {
Complex c;
c.real = c1.real - c2.real;
c.imag = c1.imag - c2.imag;
return c;
}
```
在复数Complex类中,我们重载了乘法运算符"*"和除法运算符"/",并且使用了友元函数重载加法运算符"+"和减法运算符"-"。
下面是一个使用复数Complex类的例子:
```cpp
int main() {
Complex c1(1, 2);
Complex c2(2, -3);
Complex c3 = c1 * c2;
c3.display(); // 显示:8 - 1i
Complex c4 = c1 / c2;
c4.display(); // 显示:-0.4 + 0.8i
Complex c5 = c1 + c2;
c5.display(); // 显示:3 - 1i
Complex c6 = c1 - c2;
c6.display(); // 显示:-1 + 5i
return 0;
}
```
在这个例子中,我们创建了两个复数对象c1和c2,分别表示1+2i和2-3i。然后我们使用乘法运算符"*"和除法运算符"/"来计算c1和c2的乘积和商,并将结果存储在c3和c4中。最后,我们使用加法运算符"+"和减法运算符"-"来计算c1和c2的和与差,并将结果存储在c5和c6中。最后,我们调用display()函数来显示每个复数对象的值。
希望这个例子可以帮助你了解如何使用复数类。
阅读全文
相关推荐
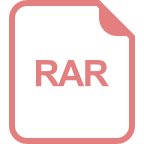





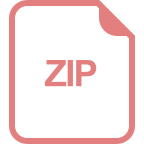
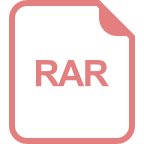
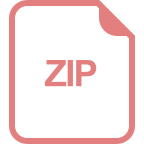
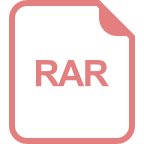
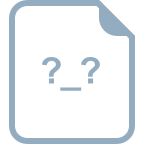
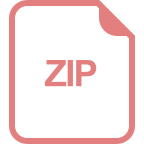
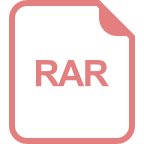