class SelfAttention(nn.Module): def init(self, in_channels, reduction=4): super(SelfAttention, self).init() self.avg_pool = nn.AdaptiveAvgPool2d((1, 1)) self.fc1 = nn.Conv2d(in_channels, in_channels // reduction, 1, bias=False) self.relu = nn.ReLU(inplace=True) self.fc2 = nn.Conv2d(in_channels // reduction, in_channels, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): # print("x=", x) b, c, n = x.size() y = self.avg_pool(x) y = self.fc1(y) y = self.relu(y) y = self.fc2(y) y = self.sigmoid(y) return x * y.expand_as(x)这是我的自注意力模块,却提示我: File "/root/autodl-tmp/project/tools/../lib/net/pointnet2_msg.py", line 91, in forward y = self.fc1(y) File "/root/miniconda3/lib/python3.8/site-packages/torch/nn/modules/module.py", line 727, in _call_impl result = self.forward(*input, **kwargs) File "/root/miniconda3/lib/python3.8/site-packages/torch/nn/modules/conv.py", line 423, in forward return self._conv_forward(input, self.weight) File "/root/miniconda3/lib/python3.8/site-packages/torch/nn/modules/conv.py", line 419, in _conv_forward return F.conv2d(input, weight, self.bias, self.stride, RuntimeError: Expected 4-dimensional input for 4-dimensional weight [256, 1024, 1, 1], but got 3-dimensional input of size [16, 1, 1] instead,你知道是为什么吗?该如何解决?你可以给我提供详细的解决代码和注释吗?
时间: 2023-11-19 16:05:56 浏览: 121
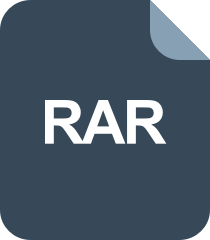
lll.rar_LMSprimsp.wav_lll_noise reduction _函数降噪_降噪
这个错误提示说期望输入是4维的,但你提供的是3维的。这是因为在你的自注意力模块中,输入 `x` 的维度是 `(batch_size, channels, n)`,而在执行 `y = self.avg_pool(x)` 操作后,`y` 的维度会变成 `(batch_size, channels, 1, 1)`,这时你需要将 `y` 的维度再变回 `(batch_size, channels, n)`。可以使用 `torch.squeeze()` 函数来实现这个操作。
解决代码如下:
```
class SelfAttention(nn.Module):
def __init__(self, in_channels, reduction=4):
super(SelfAttention, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool2d((1, 1))
self.fc1 = nn.Conv2d(in_channels, in_channels // reduction, 1, bias=False)
self.relu = nn.ReLU(inplace=True)
self.fc2 = nn.Conv2d(in_channels // reduction, in_channels, 1, bias=False)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
b, c, n = x.size()
y = self.avg_pool(x)
y = self.fc1(y)
y = self.relu(y)
y = self.fc2(y)
y = self.sigmoid(y)
# 将 y 的维度变回 (batch_size, channels, n)
y = y.squeeze(dim=-1).squeeze(dim=-1)
return x * y.unsqueeze(dim=-1).expand_as(x)
```
注释已经加上了,希望能够帮到你。
阅读全文
相关推荐
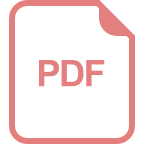
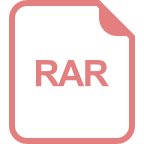








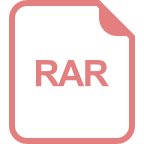
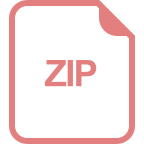
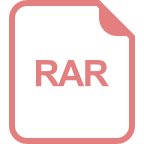
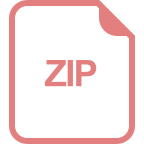
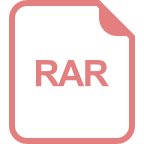
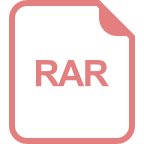
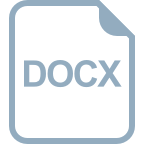