【问题描述】假设一个算术表达式的所有操作数都是10以内的整数,请将该表达式转换为后缀表达式。 【输入形式】第一行输入一个中缀表达式字符串 【输出形式】第二行输出后缀表达式 【样例输入】4*3/(2-5)*(2+3) 【样例输出】43*25-/23+*
时间: 2023-12-04 13:04:59 浏览: 35
思路:
1. 建立一个操作符栈和一个结果队列。
2. 遍历中缀表达式,遇到数字直接加入结果队列。
3. 遇到操作符,判断当前操作符与操作符栈顶操作符的优先级,如果当前操作符的优先级小于或等于栈顶操作符的优先级,则将栈顶操作符出栈并加入结果队列,直到栈顶操作符的优先级小于当前操作符的优先级或者栈为空,然后将当前操作符入栈。
4. 遍历完成后,将操作符栈中的所有操作符依次弹出并加入结果队列中。
5. 最终结果队列中的元素就是后缀表达式。
代码:
相关问题
写出符合以下要求的代码【问题描述】假设一个算术表达式的所有操作数都是10以内的整数,请将该表达式转换为后缀表达式。 【输入形式】第一行输入一个中缀表达式字符串 【输出形式】第二行输出后缀表达式 【样例输入】4*3/(2-5)*(2+3) 【样例输出】43*25-/23+*
```python
# 定义操作符优先级
priority = {'(': 0, '+': 1, '-': 1, '*': 2, '/': 2}
# 中缀表达式转后缀表达式
def infix_to_postfix(infix):
postfix = [] # 用列表存储后缀表达式
stack = [] # 用栈辅助转换
for c in infix:
if c.isdigit(): # 如果是数字,直接加入后缀表达式
postfix.append(c)
elif c == '(': # 如果是左括号,入栈
stack.append(c)
elif c == ')': # 如果是右括号,将栈中左括号之前的运算符全部弹出并加入后缀表达式
while stack[-1] != '(':
postfix.append(stack.pop())
stack.pop() # 弹出左括号
else: # 如果是运算符
while stack and priority[c] <= priority.get(stack[-1], 0): # 如果栈不为空且栈顶运算符优先级大于等于当前运算符
postfix.append(stack.pop()) # 将栈顶元素弹出并加入后缀表达式
stack.append(c) # 当前运算符入栈
while stack: # 将栈中剩余的运算符全部弹出并加入后缀表达式
postfix.append(stack.pop())
return ''.join(postfix) # 将列表转成字符串输出
# 测试代码
infix = input().strip()
postfix = infix_to_postfix(infix)
print(postfix)
```
用c语言写出符合以下要求的代码【问题描述】假设一个算术表达式的所有操作数都是10以内的整数,请将该表达式转换为后缀表达式。 【输入形式】第一行输入一个中缀表达式字符串 【输出形式】第二行输出后缀表达式 【样例输入】43/(2-5)(2+3) 【样例输出】4325-/23+
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_EXPR_LEN 100
#define MAX_STACK_SIZE 100
typedef struct {
int top;
char data[MAX_STACK_SIZE];
} Stack;
void initStack(Stack *stack) {
stack->top = -1;
}
int isEmpty(Stack *stack) {
return stack->top == -1;
}
int isFull(Stack *stack) {
return stack->top == MAX_STACK_SIZE - 1;
}
void push(Stack *stack, char c) {
if (isFull(stack)) {
printf("stack is full\n");
return;
}
stack->data[++stack->top] = c;
}
char pop(Stack *stack) {
if (isEmpty(stack)) {
printf("stack is empty\n");
return '\0';
}
return stack->data[stack->top--];
}
char top(Stack *stack) {
if (isEmpty(stack)) {
printf("stack is empty\n");
return '\0';
}
return stack->data[stack->top];
}
int isDigit(char c) {
return c >= '0' && c <= '9';
}
int priority(char op) {
switch (op) {
case '(':
return 1;
case '+':
case '-':
return 2;
case '*':
case '/':
return 3;
default:
return 0;
}
}
void infixToPostfix(char *expr, char *postfix) {
Stack stack;
initStack(&stack);
int len = strlen(expr);
int i, j;
char c;
for (i = 0, j = 0; i < len; i++) {
c = expr[i];
if (isDigit(c)) {
postfix[j++] = c;
} else if (c == '(') {
push(&stack, c);
} else if (c == ')') {
while (top(&stack) != '(') {
postfix[j++] = pop(&stack);
}
pop(&stack);
} else {
while (!isEmpty(&stack) && priority(c) <= priority(top(&stack))) {
postfix[j++] = pop(&stack);
}
push(&stack, c);
}
}
while (!isEmpty(&stack)) {
postfix[j++] = pop(&stack);
}
postfix[j] = '\0';
}
int main() {
char infix[MAX_EXPR_LEN], postfix[MAX_EXPR_LEN];
scanf("%s", infix);
infixToPostfix(infix, postfix);
printf("%s\n", postfix);
return 0;
}
相关推荐
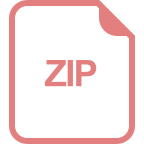
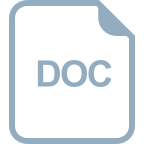
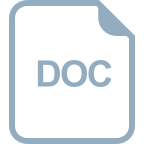










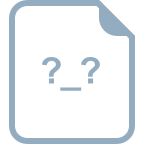
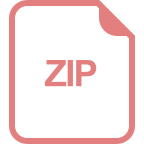
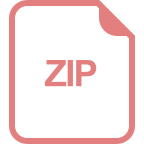