帮我写一段K Line通信协议的通信代码
时间: 2024-05-09 13:14:50 浏览: 12
K Line通信协议是一种用于汽车诊断的串行通信协议,下面是一个简单的K Line通信协议通信代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <termios.h>
#include <fcntl.h>
int main(int argc, char *argv[])
{
int fd = 0;
struct termios opt;
char buf[256] = {0};
int len = 0;
// 打开串口
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open ttyS0 failed");
return -1;
}
// 配置串口参数
memset(&opt, 0, sizeof(opt));
cfsetispeed(&opt, B9600);
cfsetospeed(&opt, B9600);
opt.c_cflag &= ~CSIZE;
opt.c_cflag |= CS8;
opt.c_cflag &= ~PARENB;
opt.c_cflag &= ~CSTOPB;
opt.c_cflag &= ~CRTSCTS;
opt.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
opt.c_iflag &= ~(IXON | IXOFF | IXANY);
opt.c_iflag &= ~(INLCR | ICRNL | IGNCR);
opt.c_oflag &= ~(ONLCR | OCRNL);
opt.c_cc[VTIME] = 0;
opt.c_cc[VMIN] = 1;
tcsetattr(fd, TCSANOW, &opt);
// 发送K Line命令
buf[0] = 0x80; // 保留位
buf[1] = 0xF1; // 服务ID
buf[2] = 0x10; // 数据长度
buf[3] = 0x81; // 模式
buf[4] = 0x12; // 检查和
len = write(fd, buf, 5);
if (len != 5) {
perror("write failed");
return -1;
}
// 读取K Line命令响应
memset(buf, 0, sizeof(buf));
len = read(fd, buf, sizeof(buf));
if (len <= 0) {
perror("read failed");
return -1;
}
// 打印响应结果
for (int i = 0; i < len; i++) {
printf("%02X ", buf[i]);
}
printf("\n");
// 关闭串口
close(fd);
return 0;
}
```
这个代码示例中,首先打开了串口设备`/dev/ttyS0`,然后配置了串口参数,接着发送了一个K Line命令,最后读取并打印了K Line命令的响应结果。你可以根据自己的具体需求进行修改和优化。
相关推荐
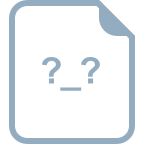
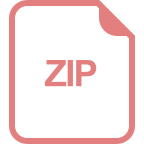
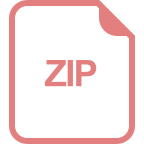














