用vs写一段解析DBC的程序
时间: 2023-11-01 15:06:39 浏览: 82
DBC是一种CAN网络通信协议,在使用CAN通信时需要解析DBC文件。以下是一个使用Visual Studio编写的解析DBC文件的示例程序:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
struct DBCMessage {
string name;
int id;
vector<string> signals;
};
struct DBCSignal {
string name;
int start_bit;
int bit_length;
double factor;
double offset;
double min_value;
double max_value;
string unit;
};
int main() {
// 打开DBC文件
ifstream file("example.dbc");
if (!file.is_open()) {
cout << "Error: failed to open file." << endl;
return 1;
}
// 解析DBC文件
vector<DBCMessage> messages;
vector<DBCSignal> signals;
string line;
while (getline(file, line)) {
// 解析消息定义
if (line.substr(0, 3) == "BO_") {
size_t pos1 = line.find(' ');
size_t pos2 = line.find(':', pos1);
size_t pos3 = line.find('\"', pos2);
size_t pos4 = line.find('\"', pos3 + 1);
int id = stoi(line.substr(pos1 + 1, pos2 - pos1 - 1));
string name = line.substr(pos3 + 1, pos4 - pos3 - 1);
messages.push_back({name, id, {}});
}
// 解析信号定义
else if (line.substr(0, 3) == "SG_") {
size_t pos1 = line.find(' ');
size_t pos2 = line.find(':', pos1);
size_t pos3 = line.find('|', pos2);
size_t pos4 = line.find('@', pos3);
size_t pos5 = line.find('+', pos4);
size_t pos6 = line.find('-', pos5);
size_t pos7 = line.find('[', pos6);
size_t pos8 = line.find(']', pos7);
size_t pos9 = line.find('\"', pos8);
size_t pos10 = line.find('\"', pos9 + 1);
string name = line.substr(pos1 + 1, pos2 - pos1 - 1);
int start_bit = stoi(line.substr(pos3 + 1, pos4 - pos3 - 1));
int bit_length = stoi(line.substr(pos4 + 1, pos5 - pos4 - 1));
double factor = stod(line.substr(pos5 + 1, pos6 - pos5 - 1));
double offset = stod(line.substr(pos6 + 1, pos7 - pos6 - 1));
double min_value = stod(line.substr(pos7 + 1, pos8 - pos7 - 1));
double max_value = stod(line.substr(pos8 + 1, pos9 - pos8 - 1));
string unit = line.substr(pos9 + 1, pos10 - pos9 - 1);
signals.push_back({name, start_bit, bit_length, factor, offset, min_value, max_value, unit});
messages.back().signals.push_back(name);
}
}
// 输出解析结果
for (auto& message : messages) {
cout << "Message: " << message.name << " (ID: " << message.id << ")" << endl;
for (auto& signal_name : message.signals) {
for (auto& signal : signals) {
if (signal.name == signal_name) {
cout << " Signal: " << signal.name << " (start bit: " << signal.start_bit;
cout << ", length: " << signal.bit_length << ", factor: " << signal.factor;
cout << ", offset: " << signal.offset << ", min value: " << signal.min_value;
cout << ", max value: " << signal.max_value << ", unit: " << signal.unit << ")" << endl;
}
}
}
}
return 0;
}
```
该程序首先打开DBC文件,然后按行读取文件内容,并根据行内容解析消息定义和信号定义。程序使用两个结构体分别表示消息和信号,其中消息包含名称、ID和包含的信号列表,信号包含名称、起始位、长度、因子、偏移量、最小值、最大值和单位。程序最后输出解析结果,包括每个消息的名称、ID和包含的信号列表,以及每个信号的名称、起始位、长度、因子、偏移量、最小值、最大值和单位。
注意,此示例程序仅适用于包含消息定义和信号定义的DBC文件,如果文件还包含其他信息,则需要进行相应修改。
阅读全文
相关推荐
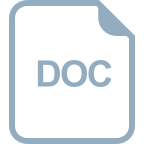
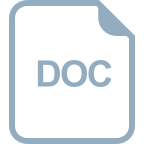
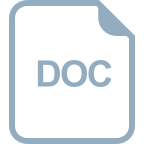


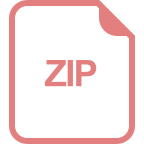
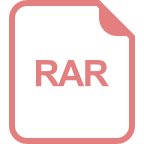
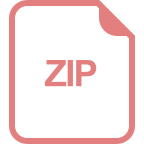
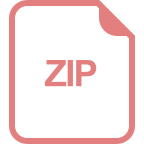
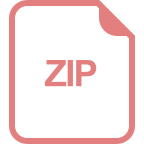
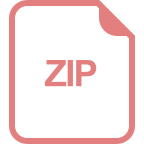
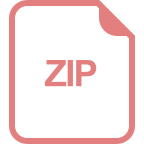
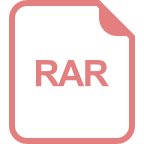
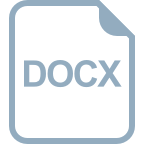
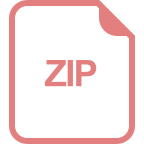
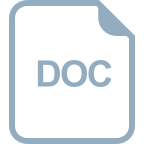
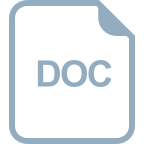
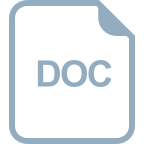
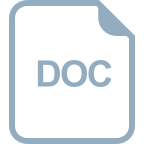