用python对一些淘宝商品信息进行爬取并避免反爬
时间: 2023-07-19 07:50:58 浏览: 92
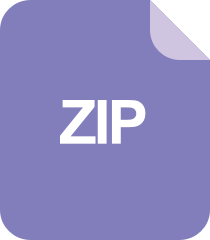
使用python对淘宝商品信息数据进行爬取

好的,针对淘宝的反爬机制,您可以采取以下几种方案:
1. 使用代理IP:通过使用代理IP,可以隐藏您的真实IP地址,降低被封禁的概率。您可以使用第三方代理IP服务商提供的API,或者自己搭建代理IP池。
以下是一个使用代理IP池的示例代码:
```python
import requests
from bs4 import BeautifulSoup
import random
# 代理IP池
proxies = [
'http://12.34.56.78:8080',
'http://23.45.67.89:8080',
'http://34.56.78.90:8080'
]
# 随机选择一个代理IP
proxy = random.choice(proxies)
url = 'https://s.taobao.com/search?q=python'
# 发送请求并获取响应
response = requests.get(url, proxies={"http": proxy})
# 解析HTML页面
soup = BeautifulSoup(response.text, 'html.parser')
# 获取所有商品信息
items = soup.find_all('div', {'class': 'item'})
# 遍历所有商品信息并输出
for item in items:
print(item.find('div', {'class': 'title'}).text.strip())
print(item.find('div', {'class': 'price'}).text.strip())
print(item.find('div', {'class': 'deal-cnt'}).text.strip())
print(item.find('a', {'class': 'J_ClickStat'}).get('href'))
```
2. 降低爬取频率:通过降低爬取频率,可以模拟人的行为,降低被封禁的概率。您可以在发送请求时设置一个随机的时间间隔,或者使用定时任务进行爬取。
以下是一个使用随机时间间隔的示例代码:
```python
import requests
from bs4 import BeautifulSoup
import time
import random
url = 'https://s.taobao.com/search?q=python'
# 循环爬取
while True:
# 发送请求并获取响应
response = requests.get(url)
# 解析HTML页面
soup = BeautifulSoup(response.text, 'html.parser')
# 获取所有商品信息
items = soup.find_all('div', {'class': 'item'})
# 遍历所有商品信息并输出
for item in items:
print(item.find('div', {'class': 'title'}).text.strip())
print(item.find('div', {'class': 'price'}).text.strip())
print(item.find('div', {'class': 'deal-cnt'}).text.strip())
print(item.find('a', {'class': 'J_ClickStat'}).get('href'))
# 休眠一段随机时间
time.sleep(random.randint(1, 5))
```
3. 使用Headless浏览器:通过使用Headless浏览器(如Selenium),可以模拟用户在浏览器中的行为,降低被封禁的概率。在使用Headless浏览器时,需要设置一些参数,如User-Agent、Cookie等,以避免被识别为爬虫。
以下是一个使用Selenium的示例代码:
```python
from selenium import webdriver
from bs4 import BeautifulSoup
url = 'https://s.taobao.com/search?q=python'
# 设置Chrome浏览器的参数
options = webdriver.ChromeOptions()
options.add_argument('--headless')
options.add_argument('--disable-gpu')
options.add_argument('--no-sandbox')
options.add_argument('--disable-dev-shm-usage')
options.add_argument('user-agent=Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3')
# 启动Chrome浏览器
driver = webdriver.Chrome(options=options)
# 发送请求并获取响应
driver.get(url)
response = driver.page_source
# 解析HTML页面
soup = BeautifulSoup(response, 'html.parser')
# 获取所有商品信息
items = soup.find_all('div', {'class': 'item'})
# 遍历所有商品信息并输出
for item in items:
print(item.find('div', {'class': 'title'}).text.strip())
print(item.find('div', {'class': 'price'}).text.strip())
print(item.find('div', {'class': 'deal-cnt'}).text.strip())
print(item.find('a', {'class': 'J_ClickStat'}).get('href'))
# 关闭Chrome浏览器
driver.quit()
```
综上,以上是三种针对淘宝反爬机制的方案,您可以根据实际情况选择其中的一种或多种来进行爬取。
阅读全文
相关推荐
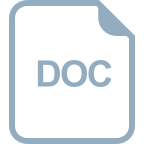
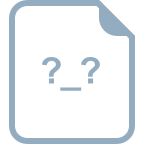















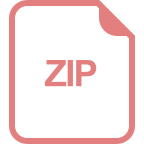