WPF datagrid 没有RowIndex属性
时间: 2023-11-21 08:04:36 浏览: 184
是的,WPF中的DataGrid控件也没有名为RowIndex的属性。但是你可以通过以下两种方式来获取当前选中行的索引:
1. 使用DataGrid控件的SelectedIndex属性。该属性表示当前选中行的索引,如下所示:
```csharp
int rowIndex = dataGrid1.SelectedIndex;
```
需要注意的是,如果用户没有选中任何行,SelectedIndex属性的值将为-1。
2. 使用DataGrid控件的SelectedItem属性和Items集合的IndexOf方法。SelectedItem属性表示当前选中行所对应的数据项,而Items集合表示DataGrid控件所绑定的数据源。因此,可以通过IndexOf方法获取当前选中行所在位置的索引,如下所示:
```csharp
int rowIndex = dataGrid1.Items.IndexOf(dataGrid1.SelectedItem);
```
需要注意的是,如果SelectedItem属性的值为null,IndexOf方法将会返回-1,表示当前没有选中任何行。
相关问题
WPF datagrid添加序号
你可以在WPF中的DataGrid中添加一个列来显示序号。以下是一些示例代码:
1. 在XAML中添加以下代码
```xaml
<DataGrid AutoGenerateColumns="False">
<DataGrid.Columns>
<DataGridTextColumn Header="#" Binding="{Binding Path=RowIndex,
RelativeSource={RelativeSource AncestorType=DataGridRow},
Converter={StaticResource RowToIndexConverter}}">
</DataGridTextColumn>
<DataGridTextColumn Header="Name" Binding="{Binding Name}"/>
<DataGridTextColumn Header="Age" Binding="{Binding Age}"/>
</DataGrid.Columns>
</DataGrid>
```
2. 创建一个名为 "RowToIndexConverter" 的转换器
```csharp
public class RowToIndexConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
DataGridRow row = value as DataGridRow;
if (row != null)
return row.GetIndex() + 1;
else
return 0;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
```
这段代码将在DataGrid中添加一个列来显示序号,并使用 "RowToIndexConverter" 转换器来将行索引转换为序号。
wpf datagrid 拖动调序
WPF的DataGrid控件可以很方便地实现拖动调序的功能。下面我将详细解释如何在WPF中实现这个功能。
首先,我们需要将DataGrid控件的CanUserSortColumns属性设置为false,以禁止用户通过点击表头来排序列。然后,我们需要为DataGrid控件的PreviewMouseLeftButtonDown、PreviewMouseMove和PreviewMouseLeftButtonUp事件编写事件处理程序。
在PreviewMouseLeftButtonDown事件处理程序中,我们需要记录鼠标按下时所点击的行,并保存其索引。代码如下:
```
private int rowIndex = -1;
private void dataGrid_PreviewMouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
DataGridRow row = VisualTreeHelper.GetParent(e.OriginalSource as DependencyObject) as DataGridRow;
if (row != null)
{
rowIndex = dataGrid.Items.IndexOf(row.Item);
}
}
```
在PreviewMouseMove事件处理程序中,我们需要判断是否进行了拖动操作,并设置DataGrid控件的AllowDrop属性为true。然后,我们需要使用DragDrop.DoDragDrop方法来启动拖动操作。代码如下:
```
private void dataGrid_PreviewMouseMove(object sender, MouseEventArgs e)
{
if (rowIndex >= 0 && e.LeftButton == MouseButtonState.Pressed)
{
DataGridRow row = VisualTreeHelper.GetParent(e.OriginalSource as DependencyObject) as DataGridRow;
if (row != null && row.Item != null)
{
DragDrop.DoDragDrop(dataGrid, row.Item, DragDropEffects.Move);
}
}
}
```
最后,在PreviewMouseLeftButtonUp事件处理程序中,我们需要根据拖动操作的目标行来调整数据源中行的顺序。代码如下:
```
private void dataGrid_PreviewMouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
if (rowIndex >= 0 && e.LeftButton == MouseButtonState.Released)
{
DataGridRow row = VisualTreeHelper.GetParent(e.OriginalSource as DependencyObject) as DataGridRow;
if (row != null)
{
int newIndex = dataGrid.Items.IndexOf(row.Item);
if (newIndex >= 0 && newIndex != rowIndex)
{
// 调整数据源中行的顺序
// 注意:需要使用ObservableCollection<T>作为数据源类型,以便自动更新DataGrid控件的显示
}
}
rowIndex = -1;
}
}
```
以上就是实现WPF DataGrid拖动调序的基本步骤。需要注意的是,调整数据源中行的顺序时,我们需要使用ObservableCollection<T>作为数据源类型,以便自动更新DataGrid控件的显示。
阅读全文
相关推荐
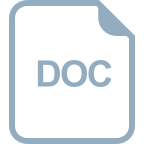
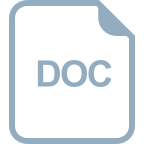
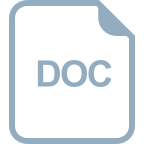
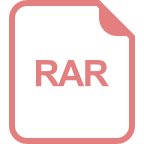



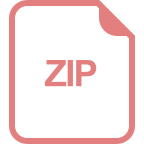







