class Point { public: Point(int x = 0, int y = 0) : m_x(x), m_y(y) {} ~Point() {} double Distance(Point p) { return sqrt((m_x - p.m_x) * (m_x - p.m_x) + (m_y - p.m_y) * (m_y - p.m_y)); } protected: int m_x, m_y; }; class Rectangle : public Point { public: Rectangle(int x1, int y1, int x2, int y2) : p1(x1, y1), p2(x2, y2) {} ~Rectangle(){} double Area() { p1.Distance(Point(p1.m_x, p2.m_y)) * p1.Distance(Point(p2.m_x, p1.m_y)); } private: Point p1, p2; };无法访问 protected 成员
时间: 2023-12-15 20:04:50 浏览: 441
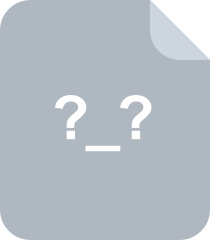
Point.class
在这段代码中,类 `Point` 中的 `m_x` 和 `m_y` 是被声明为 `protected` 的,因此派生类 `Rectangle` 可以访问这些成员。但是在 `Rectangle` 类的 `Area()` 函数中,访问 `Point` 对象的成员变量时却使用了 `p1.m_x` 和 `p2.m_y` 的形式,这是错误的。应该使用 `p1.Distance(Point(p1.m_x, p2.m_y))` 和 `p1.Distance(Point(p2.m_x, p1.m_y))` 计算矩形的面积,即先通过 `Point` 类的 `Distance()` 函数计算出矩形的对角线长度,再根据对角线长度计算面积,代码如下:
```cpp
class Point {
public:
Point(int x = 0, int y = 0) : m_x(x), m_y(y) {}
~Point() {}
double Distance(Point p) {
return sqrt((m_x - p.m_x) * (m_x - p.m_x) + (m_y - p.m_y) * (m_y - p.m_y));
}
protected:
int m_x, m_y;
};
class Rectangle : public Point {
public:
Rectangle(int x1, int y1, int x2, int y2) : p1(x1, y1), p2(x2, y2) {}
~Rectangle(){}
double Area() {
return p1.Distance(Point(p1.m_x, p2.m_y)) * p1.Distance(Point(p2.m_x, p1.m_y));
}
private:
Point p1, p2;
};
```
阅读全文
相关推荐
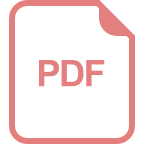
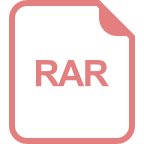












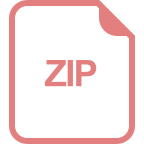