具体的子任务: a) 定义一个名为 Question 的类来保存单个问题,即文本、可能的答案和正确答案索引; (0.25P) b) 编写一个方法,通过 JFileChooser 选择文件,并将该文件中的所有问题读取到 Question 对象的数组/列表中(假设该文件具有上述结构); (0.25点) c) 使用上述组件设计和实现 GUI:菜单、显示问题和答案的能力、选择答案、显示结果和分数以及继续下一个问题的能力。 (适当的布局:1P,类扩展JFrame:0.25P, 类遵循OOP原则:0.25P,主方法全局设置:0.25P) d) 编写一个方法以在您设计的 GUI 上显示问题; (0.25点) e) 实现一个 actionPerforming 方法来响应用户与 GUI 的交互。确保根据需要启用和禁用交互式组件,例如,用户不应在没有先选择答案的情况下跳到下一个问题,并且他们不应能够在完成当前测验之前加载新测验; (类实现 ActionListener:0.25P,启用/禁用组件:0.25P, 按要求工作:1P) 可选挑战:随机化问题和答案的显示顺序。问题:你怎么知道哪个答案是正确的?选择答案后,如果用户选择了正确答案,请以绿色突出显示。如果他们选择了错误的答案,请以红色突出显示他们的答案,以绿色突出显示正确的答案。单击“下一步”时,重置颜色。 添加一个拨号框,确认用户在按菜单中的“退出”时是否确实要退出程序。 可能的最终设计 从左上到右下,启动程序,加载问题文件,选择正确答案(粉红色),单击下一步,选择错误答案(6),单击下一步,游戏结束。
时间: 2024-02-09 17:09:41 浏览: 24
这是一项比较复杂的任务,需要考虑多个方面。下面我将列出一些实现思路,供您参考:
a)定义一个名为 Question 的类来保存单个问题,即文本、可能的答案和正确答案索引。
```java
public class Question {
private String questionText;
private String[] answerOptions;
private int correctAnswerIndex;
public Question(String questionText, String[] answerOptions, int correctAnswerIndex) {
this.questionText = questionText;
this.answerOptions = answerOptions;
this.correctAnswerIndex = correctAnswerIndex;
}
public String getQuestionText() {
return questionText;
}
public String[] getAnswerOptions() {
return answerOptions;
}
public int getCorrectAnswerIndex() {
return correctAnswerIndex;
}
}
```
b)编写一个方法,通过 JFileChooser 选择文件,并将该文件中的所有问题读取到 Question 对象的数组/列表中。
```java
public List<Question> readQuestionsFromFile() {
List<Question> questionList = new ArrayList<>();
JFileChooser fileChooser = new JFileChooser();
fileChooser.showOpenDialog(null);
File file = fileChooser.getSelectedFile();
try (BufferedReader reader = new BufferedReader(new FileReader(file))) {
String line;
while ((line = reader.readLine()) != null) {
String[] questionData = line.split(",");
String questionText = questionData[0];
String[] answerOptions = Arrays.copyOfRange(questionData, 1, questionData.length - 1);
int correctAnswerIndex = Integer.parseInt(questionData[questionData.length - 1]);
Question question = new Question(questionText, answerOptions, correctAnswerIndex);
questionList.add(question);
}
} catch (IOException e) {
e.printStackTrace();
}
return questionList;
}
```
c)使用上述组件设计和实现 GUI:菜单、显示问题和答案的能力、选择答案、显示结果和分数以及继续下一个问题的能力。
在设计 GUI 时,可以参考以下代码:
```java
public class QuizApp extends JFrame implements ActionListener {
private JLabel questionLabel;
private JRadioButton[] answerButtons;
private JButton nextButton;
private int currentQuestionIndex;
private int score;
private List<Question> questionList;
public QuizApp() {
super("Quiz App");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
// 添加菜单
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("File");
JMenuItem exitMenuItem = new JMenuItem("Exit");
exitMenuItem.addActionListener(this);
fileMenu.add(exitMenuItem);
menuBar.add(fileMenu);
setJMenuBar(menuBar);
// 添加问题和答案
JPanel questionPanel = new JPanel(new BorderLayout());
questionLabel = new JLabel("");
questionPanel.add(questionLabel, BorderLayout.NORTH);
JPanel answerPanel = new JPanel(new GridLayout(0, 1));
answerButtons = new JRadioButton[4];
ButtonGroup answerButtonGroup = new ButtonGroup();
for (int i = 0; i < 4; i++) {
answerButtons[i] = new JRadioButton("");
answerButtons[i].addActionListener(this);
answerButtonGroup.add(answerButtons[i]);
answerPanel.add(answerButtons[i]);
}
questionPanel.add(answerPanel, BorderLayout.CENTER);
add(questionPanel, BorderLayout.CENTER);
// 添加“下一步”按钮
nextButton = new JButton("Next");
nextButton.addActionListener(this);
add(nextButton, BorderLayout.SOUTH);
// 初始化问题列表和当前问题索引
questionList = readQuestionsFromFile();
currentQuestionIndex = 0;
// 显示第一个问题
showQuestion(currentQuestionIndex);
pack();
setVisible(true);
}
private void showQuestion(int questionIndex) {
Question question = questionList.get(questionIndex);
questionLabel.setText(question.getQuestionText());
String[] answerOptions = question.getAnswerOptions();
for (int i = 0; i < answerButtons.length; i++) {
JRadioButton answerButton = answerButtons[i];
if (i < answerOptions.length) {
answerButton.setText(answerOptions[i]);
answerButton.setEnabled(true);
} else {
answerButton.setText("");
answerButton.setEnabled(false);
}
}
clearAnswerSelection();
}
private void clearAnswerSelection() {
for (JRadioButton answerButton : answerButtons) {
answerButton.setSelected(false);
answerButton.setBackground(null);
}
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() instanceof JRadioButton) {
JRadioButton selectedButton = (JRadioButton) e.getSource();
int selectedAnswerIndex = -1;
for (int i = 0; i < answerButtons.length; i++) {
if (selectedButton == answerButtons[i]) {
selectedAnswerIndex = i;
break;
}
}
if (selectedAnswerIndex == questionList.get(currentQuestionIndex).getCorrectAnswerIndex()) {
selectedButton.setBackground(Color.GREEN);
score++;
} else {
selectedButton.setBackground(Color.RED);
answerButtons[questionList.get(currentQuestionIndex).getCorrectAnswerIndex()].setBackground(Color.GREEN);
}
} else if (e.getSource() == nextButton) {
if (currentQuestionIndex < questionList.size() - 1) {
currentQuestionIndex++;
showQuestion(currentQuestionIndex);
} else {
JOptionPane.showMessageDialog(this, "Game over! Your score is " + score);
clearAnswerSelection();
nextButton.setEnabled(false);
for (JRadioButton answerButton : answerButtons) {
answerButton.setEnabled(false);
}
}
} else if (e.getActionCommand().equals("Exit")) {
int result = JOptionPane.showConfirmDialog(this, "Are you sure you want to exit?");
if (result == JOptionPane.YES_OPTION) {
System.exit(0);
}
}
}
public static void main(String[] args) {
QuizApp quizApp = new QuizApp();
}
}
```
d)编写一个方法以在您设计的 GUI 上显示问题。
在上面的代码中,showQuestion() 方法用于在 GUI 上显示问题和答案。
e)实现一个 actionPerforming 方法来响应用户与 GUI 的交互。
在上面的代码中,actionPerformed() 方法用于处理用户与 GUI 的交互。在该方法中根据用户的操作类型执行相应的操作,例如选择答案、点击“下一步”等。同时,需要根据用户的选择改变 GUI 上的状态,例如设置按钮的颜色、禁用/启用按钮等。
相关推荐
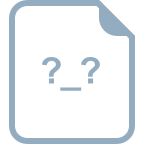
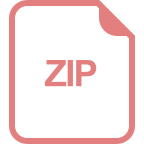















