输入一组字符(以#号结尾),对该组字符做一个统计,统计字母、数字和其它字符的 个数,输出统计结果,编写程序要求使用while语句
时间: 2024-03-17 18:39:52 浏览: 20
好的,以下是使用C++语言编写的程序:
```
#include <iostream>
using namespace std;
int main()
{
char ch; // 定义一个字符变量
int letter = 0, digit = 0, other = 0; // 初始化字母、数字和其他字符的计数器
cout << "请输入一组字符(以#号结尾):\n";
while ((ch = cin.get()) != '#') // 循环读取字符,直到读取到#号为止
{
if (isalpha(ch)) // 判断是否为字母
letter++;
else if (isdigit(ch)) // 判断是否为数字
digit++;
else // 其他字符
other++;
}
cout << "字母个数:" << letter << endl;
cout << "数字个数:" << digit << endl;
cout << "其他字符个数:" << other << endl;
return 0;
}
```
程序运行示例:
```
请输入一组字符(以#号结尾):
Hello, world! 123#
字母个数:12
数字个数:3
其他字符个数:2
```
相关问题
用C语言输入一组字符(以#号结尾),对该组字符做一个统计,统计字母、数字和其它字符的 个数,输出统计结果,编写程序要求使用while语句
好的,以下是使用C语言编写的程序:
```c
#include <stdio.h>
#include <ctype.h> // 需要使用isalpha和isdigit函数
int main()
{
char ch; // 定义一个字符变量
int letter = 0, digit = 0, other = 0; // 初始化字母、数字和其他字符的计数器
printf("请输入一组字符(以#号结尾):\n");
while ((ch = getchar()) != '#') // 循环读取字符,直到读取到#号为止
{
if (isalpha(ch)) // 判断是否为字母
letter++;
else if (isdigit(ch)) // 判断是否为数字
digit++;
else // 其他字符
other++;
}
printf("字母个数:%d\n", letter);
printf("数字个数:%d\n", digit);
printf("其他字符个数:%d\n", other);
return 0;
}
```
程序运行示例:
```
请输入一组字符(以#号结尾):
Hello, world! 123#
字母个数:12
数字个数:3
其他字符个数:2
```
9. 输入一组字符(以#号结尾),对该组字符做一个统计,统计字母、数字和其它字符的个数,输出统计结果,编写程序要求使用while语句
下面是使用Python编写的程序,实现输入一组字符并统计字母、数字和其他字符的个数。
```python
letter_count = 0
digit_count = 0
other_count = 0
while True:
char = input("请输入一个字符:")
if char == "#":
break
elif char.isalpha():
letter_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print("字母个数:", letter_count)
print("数字个数:", digit_count)
print("其他字符个数:", other_count)
```
程序中,我们使用了一个while循环来不断地输入字符,当输入的字符为#时,循环结束。在循环体中,使用isalpha()函数判断输入的字符是否为字母,使用isdigit()函数判断是否为数字,否则就认为是其他字符。每次判断完后,将对应的计数器加1。最后输出各种字符的个数即可。
相关推荐
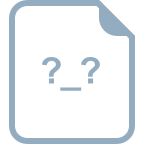












