提示错误:TypeError: Only valid with DatetimeIndex, TimedeltaIndex or PeriodIndex, but got an instance of 'Int64Index'
时间: 2024-02-14 22:17:35 浏览: 28
这个错误提示通常是因为DataFrame的索引不是时间类型的索引,而是整数类型的索引。要想使用时间相关的方法,需要先将索引转换为时间类型的索引。以下是示例代码:
```python
import pandas as pd
# 读取数据
df = pd.read_csv("data.csv")
# 将“交易日期”字段转换为时间类型,并设置为索引
df["交易日期"] = pd.to_datetime(df["交易日期"])
df = df.set_index("交易日期")
# 使用时间相关的方法
weekly_df = df.resample("W").sum()
rolling_df = df.rolling("7D").sum()
# 打印结果
print("Weekly data:")
print(weekly_df)
print("\nRolling data:")
print(rolling_df)
```
在示例代码中,我们使用`pd.to_datetime`方法将“交易日期”字段转换为时间类型,并使用`set_index`方法将其设置为索引。然后,可以使用时间相关的方法,例如`resample`方法对数据进行重采样,`rolling`方法对数据进行滚动计算。最后,使用`print`函数打印输出结果。
相关问题
TypeError: Only valid with DatetimeIndex, TimedeltaIndex or PeriodIndex, but got an instance of 'Index'、
在Python中,当对股票进行时间序列的重分类时,如果出现错误TypeError: Only valid with DatetimeIndex, TimedeltaIndex or PeriodIndex, but got an instance of 'Index',这是因为索引的数据类型不是日期时间格式。为了解决这个问题,有以下几种方法可以尝试:
1. 将日期列设置为索引:可以使用`set_index()`函数将日期列设置为索引。例如,可以使用以下代码将'Date'列设置为索引:`stock_df = pd.DataFrame(stock_df).set_index('Date')`。这样做可以将索引的数据类型转换为日期时间格式,从而解决错误。
2. 判断索引是否为时间格式:可以使用if语句判断索引是否为时间格式。如果索引不是时间格式,则需要将其转换为时间格式。可以使用`pd.to_datetime()`函数将索引转换为时间格式。例如,可以使用以下代码将索引列'time'转换为时间格式:`qihuo3['time'] = pd.to_datetime(qihuo3['time'])`。
3. 判断索引中是否有非时间格式:有时候,索引中可能包含非时间格式的值,这也会导致出现错误。可以使用条件语句来排除索引中非时间格式的行。例如,可以使用以下代码排除'time_A'或'time_B'为0的行:`qihuo3 = qihuo3[(qihuo3['time_A']!=0) & (qihuo3['time_B']!=0)]`。然后,可以使用`max()`函数取' time_A'和'time_B'中较大的时间作为索引:`qihuo3['time'] = qihuo3[['time_A','time_B']].max(axis=1)`。
通过以上方法,你可以解决TypeError: Only valid with DatetimeIndex, TimedeltaIndex or PeriodIndex, but got an instance of 'Index'的错误,并成功进行时间序列的重分类。
typeerror: only valid with datetimeindex, timedeltaindex or periodindex, but got an instance of 'index'
这个错误是因为你传入的索引不是DatetimeIndex、TimedeltaIndex或PeriodIndex类型,而是Index类型。可能是因为你在使用某些时间序列相关的函数时,传入了错误的索引类型。你需要检查一下你的代码,确保传入的索引类型正确。
相关推荐
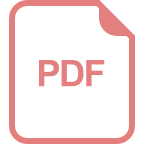
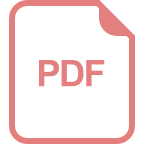








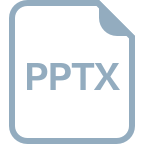
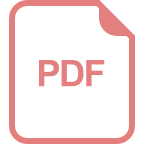