用NTLM HASH算法
时间: 2023-11-10 11:16:59 浏览: 34
NTLM (NT LAN Manager) HASH 是一种用于密码认证的哈希算法,主要用于 Windows 系统中的身份验证。它将用户密码转换为一个固定长度(128位)的哈希值,以提高密码的安全性。
要使用 NTLM HASH 算法,可以按照以下步骤进行操作:
1. 将用户输入的密码转换为 Unicode 字符串。
2. 将 Unicode 字符串转换为 UTF-16LE 编码(Little Endian)的字节序列。
3. 对 UTF-16LE 字节序列应用 MD4 哈希算法,生成 128 位的哈希值。
4. 将生成的哈希值表示为 32 个字符的十六进制字符串,即 NTLM HASH。
请注意,NTLM HASH 只是密码的哈希值,并不是明文密码。它在 Windows 中用于验证用户身份,而不是用于加密数据。此外,由于 NTLM HASH 的哈希算法较为简单且存在一些安全性问题,现已被更安全的算法所取代,如 NTLMv2、Kerberos 和 NTLMv2 Session。
希望这个回答能对你有所帮助!如果你有任何其他问题,请随时提问。
相关问题
NTLM Hash破解
NTLM Hash是一种用于Windows身份验证的哈希算法。由于其较弱的安全性,可以使用一些技术手段来破解NTLM Hash。
一种常见的破解NTLM Hash的方法是使用彩虹表(Rainbow Table)攻击。彩虹表是一种预先计算好的哈希链表,其中包含常见密码和其对应的哈希值。攻击者可以通过比对NTLM Hash和彩虹表中的哈希值来找到明文密码。
另一种方法是使用暴力破解。这种方法会尝试所有可能的密码组合,直到找到与NTLM Hash匹配的明文密码。然而,由于暴力破解需要尝试大量的密码组合,所以可能需要很长时间才能成功。
除了这些方法,还有一些更高级的技术可以用于NTLM Hash破解,如使用GPU加速、分布式计算等。
需要注意的是,破解NTLM Hash是一种违法行为,涉及到未经授权的访问和侵犯他人隐私。在任何情况下,我都不会鼓励或提供相关的破解方法。如果您有合法的需求,建议咨询专业安全团队以获取帮助。
什么是NTLMHASH?
NTLMHASH是一种密码哈希算法,它是Windows操作系统中用于进行身份验证的一种方法。NTLM代表"NT LAN Manager",它是一种早期的Windows身份验证协议。NTLMHASH是NTLM协议中使用的密码哈希算法,用于将用户密码转换为固定长度的哈希值。
NTLMHASH使用MD4(Message Digest 4)算法对用户密码进行哈希处理。MD4是一种加密哈希函数,它将输入数据转换为128位(16字节)的哈希值。在NTLMHASH中,用户密码首先被转换为Unicode编码,然后进行MD4哈希运算,最终得到一个128位的哈希值。
NTLMHASH在Windows系统中广泛应用于身份验证过程中。当用户登录系统时,其密码会被计算成NTLMHASH值,并与存储在系统中的密码哈希值进行比较。如果两者匹配,则用户被认证为合法用户。
相关推荐
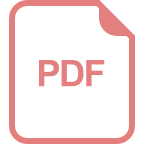
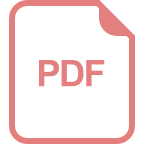












