帮我写一个仓库管理系统
时间: 2024-05-30 22:15:20 浏览: 128
好的,我可以为您提供一些基本的思路和建议来开发一个仓库管理系统。首先,您需要确定您的系统需要哪些功能和模块,如库存管理、出入库记录、统计报表等。然后,您可以选择合适的技术栈来实现您的系统。
其中,有一些关键的术语需要了解:商品、仓库、入库、出库、库存、库存记录等。在设计数据结构时,需要考虑这些术语的关系和属性,例如每个商品的名称、编号、价格、库存数量等。
对于仓库管理系统,需要有良好的界面设计和用户体验,以方便用户操作。同时,也需要考虑安全性和权限控制,如对于不同用户的操作权限进行限制等。最后,还需要进行测试和调试,确保系统功能正常运行。
当然,以上只是一个简单的建议,具体的实现细节需要根据您具体的需求和技术实现来定。如果您有具体的问题或需求,请随时与我交流。
相关问题
帮我写一个仓库管理系统Python代码
好的,以下是一个简单的仓库管理系统的Python代码,您可以根据需要进行修改和优化:
```python
# 定义产品类
class Product:
def __init__(self, id, name, quantity):
self.id = id
self.name = name
self.quantity = quantity
# 定义仓库类
class Warehouse:
def __init__(self):
self.products = []
# 添加产品
def add_product(self, product):
self.products.append(product)
# 删除产品
def remove_product(self, product_id):
for product in self.products:
if product.id == product_id:
self.products.remove(product)
# 更新产品数量
def update_quantity(self, product_id, quantity):
for product in self.products:
if product.id == product_id:
product.quantity = quantity
# 查找产品
def find_product(self, product_id):
for product in self.products:
if product.id == product_id:
return product
return None
# 显示所有产品
def show_products(self):
for product in self.products:
print(f"ID: {product.id}, Name: {product.name}, Quantity: {product.quantity}")
# 主程序
if __name__ == "__main__":
warehouse = Warehouse()
# 添加产品
product1 = Product(1, "iPhone", 100)
product2 = Product(2, "iPad", 50)
warehouse.add_product(product1)
warehouse.add_product(product2)
# 显示所有产品
warehouse.show_products()
# 更新产品数量
warehouse.update_quantity(1, 50)
# 删除产品
warehouse.remove_product(2)
# 查找产品
product = warehouse.find_product(1)
if product:
print(f"ID: {product.id}, Name: {product.name}, Quantity: {product.quantity}")
else:
print("Product not found.")
```
该代码实现了一个简单的仓库管理系统,包括添加产品、删除产品、更新产品数量、查找产品和显示所有产品等功能。您可以根据实际需求进行修改和扩展。
用python帮我写一个仓库管理系统
好的,以下是一个简单的仓库管理系统的Python代码示例:
```python
class InventoryItem:
def __init__(self, name, quantity):
self.name = name
self.quantity = quantity
class Inventory:
def __init__(self):
self.items = []
def add_item(self, name, quantity):
for item in self.items:
if item.name == name:
item.quantity += quantity
break
else:
new_item = InventoryItem(name, quantity)
self.items.append(new_item)
def remove_item(self, name, quantity):
for item in self.items:
if item.name == name:
if item.quantity >= quantity:
item.quantity -= quantity
break
else:
print("Not enough quantity to remove.")
break
else:
print("Item not found.")
def display_inventory(self):
print("Inventory:")
for item in self.items:
print(f"{item.name}: {item.quantity}")
# Example usage
inventory = Inventory()
inventory.add_item("Apple", 5)
inventory.add_item("Banana", 10)
inventory.add_item("Apple", 3)
inventory.display_inventory()
inventory.remove_item("Apple", 2)
inventory.remove_item("Grape", 1)
inventory.display_inventory()
```
该系统由两个类组成:`InventoryItem`表示一个库存条目,其中包含名称和数量属性,`Inventory`表示整个库存,其中包含一个包含所有库存条目的列表。`Inventory`类有三个主要方法:`add_item`用于添加新的库存项,如果该项已存在,则增加其数量;`remove_item`用于从库存中删除指定数量的库存项;`display_inventory`用于显示整个库存清单。
在这个简单的例子中,我们首先创建一个`Inventory`对象,然后添加三个库存项。接下来,我们显示整个库存清单,然后尝试删除两个苹果和一个葡萄。最后,我们再次显示整个库存清单以查看删除操作的结果。
阅读全文
相关推荐

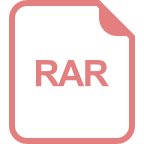











