已知有—棵以二叉链表存储的二叉树,root指向根结点,p指向二叉树中任一结点,如何求从根结点到p所指结点之间的路径?
时间: 2024-05-16 10:12:55 浏览: 6
我们可以使用递归来求解从根结点到p所指结点之间的路径。具体步骤如下:
1. 如果根结点为空或者根结点就是p结点,则直接返回根结点。
2. 递归遍历根结点的左子树,如果找到p结点,则将根结点加入路径中,并返回根结点。
3. 递归遍历根结点的右子树,如果找到p结点,则将根结点加入路径中,并返回根结点。
4. 如果左右子树中都没有找到p结点,则返回NULL。
下面是代码实现:
```
struct TreeNode {
int val;
TreeNode *left;
TreeNode *right;
TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
};
vector<TreeNode*> getPath(TreeNode* root, TreeNode* p) {
if (root == nullptr || root == p) {
vector<TreeNode*> path;
if (root == p) path.push_back(root);
return path;
}
vector<TreeNode*> leftPath = getPath(root->left, p);
if (!leftPath.empty()) {
leftPath.insert(leftPath.begin(), root);
return leftPath;
}
vector<TreeNode*> rightPath = getPath(root->right, p);
if (!rightPath.empty()) {
rightPath.insert(rightPath.begin(), root);
return rightPath;
}
return vector<TreeNode*>();
}
```
函数getPath的返回值是一个vector<TreeNode*>,表示从根结点到p结点的路径。如果路径存在,则返回路径上的所有结点,否则返回一个空vector。
相关推荐
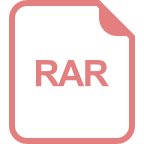
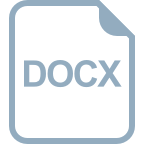
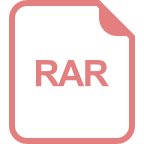














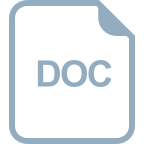