c语言中根据kruskal算法,实现求最小生成树代码
时间: 2024-03-09 14:44:59 浏览: 64
好的,以下是Kruskal算法求最小生成树的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Edge
{
int src, dest, weight;
};
struct Graph
{
int V, E;
struct Edge* edge;
};
struct Graph* create_graph(int V, int E)
{
struct Graph* graph = (struct Graph*) malloc(sizeof(struct Graph));
graph->V = V;
graph->E = E;
graph->edge = (struct Edge*) malloc(graph->E * sizeof(struct Edge));
return graph;
}
int find(int parent[], int i)
{
if (parent[i] == -1)
return i;
return find(parent, parent[i]);
}
void Union(int parent[], int x, int y)
{
int xset = find(parent, x);
int yset = find(parent, y);
parent[xset] = yset;
}
int my_comp(const void* a, const void* b)
{
struct Edge* a1 = (struct Edge*) a;
struct Edge* b1 = (struct Edge*) b;
return a1->weight > b1->weight;
}
void kruskal(struct Graph* graph)
{
int V = graph->V;
struct Edge result[V];
int e = 0;
int i = 0;
qsort(graph->edge, graph->E, sizeof(graph->edge[0]), my_comp);
int parent[V];
memset(parent, -1, sizeof(int) * V);
while (e < V - 1 && i < graph->E)
{
struct Edge next_edge = graph->edge[i++];
int x = find(parent, next_edge.src);
int y = find(parent, next_edge.dest);
if (x != y)
{
result[e++] = next_edge;
Union(parent, x, y);
}
}
printf("Following are the edges in the constructed MST\n");
int minimumCost = 0;
for (i = 0; i < e; ++i)
{
printf("%d - %d : %d\n", result[i].src, result[i].dest, result[i].weight);
minimumCost += result[i].weight;
}
printf("Minimum Cost Spanning tree : %d", minimumCost);
}
int main()
{
int V = 4;
int E = 5;
struct Graph* graph = create_graph(V, E);
graph->edge[0].src = 0;
graph->edge[0].dest = 1;
graph->edge[0].weight = 10;
graph->edge[1].src = 0;
graph->edge[1].dest = 2;
graph->edge[1].weight = 6;
graph->edge[2].src = 0;
graph->edge[2].dest = 3;
graph->edge[2].weight = 5;
graph->edge[3].src = 1;
graph->edge[3].dest = 3;
graph->edge[3].weight = 15;
graph->edge[4].src = 2;
graph->edge[4].dest = 3;
graph->edge[4].weight = 4;
kruskal(graph);
return 0;
}
```
这段代码中,我们首先定义了一个Edge结构体和Graph结构体,其中Edge结构体表示一条边,包含源节点、目标节点和权值三个属性;Graph结构体表示一个图,包含节点数、边数和边数组三个属性。然后我们定义了一些辅助函数,包括创建图、查找父节点、并查集合并、边的比较函数和Kruskal算法函数等。Kruskal算法的基本思路与前面Python代码实现中的一样,不再赘述。
阅读全文
相关推荐
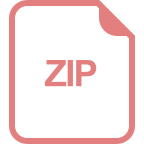


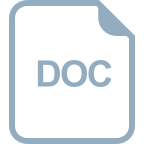
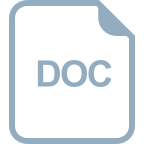
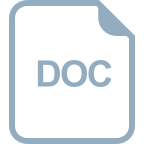
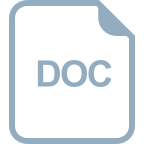
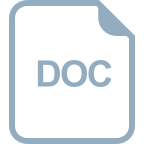
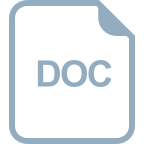




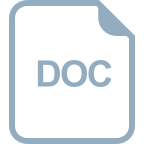


