1) 实现类Img,参考形式如下: Class Img { Img(){} void readImg(string file);//从参数file指定的路径读取图像文件,有合理的函数体即可 void writeImg(string file);//将图像数据写出到参数file指定的路径,有合理的函数体即可 enum IMG_STORE_TYPE{GRAY,GRAY_A,RGB,RGB_A;};//图像的存储形式有四种: 灰度通道,灰度通道+透明度通道,RGB通道,RGB通道+透明度通道 IMG_STORE_TYPE store_type;//根据readImg的信息设定具体的store_type。 short[] pixel_data;存储的像素颜色数据 Img UnaryOperation(ShortUnaryOperator unary_op); Img BinaryOperation(Img img, ShortBinaryOperator binary_op); }
时间: 2024-02-09 22:12:44 浏览: 74
好的,我理解了你的问题。下面是针对这个问题的回答:
首先,让我们来看一下这个类Img的定义。这个类有以下几个方法:
- Img():构造函数,用于初始化Img类的对象。
- readImg(string file):从指定路径file中读取图像文件。
- writeImg(string file):将图像数据写出到指定路径file中。
- enum IMG_STORE_TYPE:表示图像的存储形式,包括灰度通道、灰度通道+透明度通道、RGB通道和RGB通道+透明度通道。
- pixel_data:存储像素颜色数据的数组。
- UnaryOperation(ShortUnaryOperator unary_op):对图像进行一元操作,返回一个新的Img对象。
- BinaryOperation(Img img, ShortBinaryOperator binary_op):对两个图像进行二元操作,返回一个新的Img对象。
下面是这个类的具体实现:
```java
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Arrays;
import java.util.function.ShortUnaryOperator;
import java.util.function.ShortBinaryOperator;
public class Img {
private String filename;
private byte[] data;
private IMG_STORE_TYPE store_type;
private short[] pixel_data;
public Img() {
this.filename = "";
this.data = null;
this.store_type = null;
this.pixel_data = null;
}
public void readImg(String file) {
try {
this.filename = file;
this.data = Files.readAllBytes(Paths.get(file));
// Determine image store type
if (this.data.length == 3 * 256 * 256) {
this.store_type = IMG_STORE_TYPE.RGB;
} else if (this.data.length == 4 * 256 * 256) {
this.store_type = IMG_STORE_TYPE.RGB_A;
} else if (this.data.length == 256 * 256) {
this.store_type = IMG_STORE_TYPE.GRAY;
} else if (this.data.length == 2 * 256 * 256) {
this.store_type = IMG_STORE_TYPE.GRAY_A;
}
// Parse pixel data
this.pixel_data = new short[this.data.length / 2];
for (int i = 0; i < this.pixel_data.length; ++i) {
byte hi = this.data[2 * i];
byte lo = this.data[2 * i + 1];
this.pixel_data[i] = (short) ((hi << 8) | (lo & 0xff));
}
} catch (Exception e) {
System.out.println("Error reading image file: " + e.getMessage());
}
}
public void writeImg(String file) {
try {
byte[] out_data = new byte[this.data.length];
for (int i = 0; i < this.pixel_data.length; ++i) {
short pixel = this.pixel_data[i];
out_data[2 * i] = (byte) (pixel >> 8);
out_data[2 * i + 1] = (byte) (pixel & 0xff);
}
Files.write(Paths.get(file), out_data);
} catch (Exception e) {
System.out.println("Error writing image file: " + e.getMessage());
}
}
public Img UnaryOperation(ShortUnaryOperator unary_op) {
Img result = new Img();
result.filename = this.filename;
result.data = this.data.clone();
result.store_type = this.store_type;
result.pixel_data = Arrays.stream(this.pixel_data).map(unary_op).toArray();
return result;
}
public Img BinaryOperation(Img img, ShortBinaryOperator binary_op) {
Img result = new Img();
result.filename = this.filename;
result.data = this.data.clone();
result.store_type = this.store_type;
result.pixel_data = new short[this.pixel_data.length];
for (int i = 0; i < this.pixel_data.length; ++i) {
result.pixel_data[i] = binary_op.applyAsShort(this.pixel_data[i], img.pixel_data[i]);
}
return result;
}
public enum IMG_STORE_TYPE {
GRAY,
GRAY_A,
RGB,
RGB_A
}
}
```
这个实现中,我们使用了Java中的字节流和数组操作,以及Java 8中的流式操作和函数式编程。在readImg方法中,我们首先读取文件中的字节数据,并根据数据长度确定图像的存储形式。然后,我们解析像素数据,并将其存储在short类型的数组中。在writeImg方法中,我们将short类型的像素数据转换为字节数据,并将其写入文件中。在UnaryOperation方法中,我们对像素数据进行一元操作,并返回一个新的Img对象。在BinaryOperation方法中,我们对两个Img对象的像素数据进行二元操作,并返回一个新的Img对象。
希望这个回答能够解决你的问题!
阅读全文
相关推荐
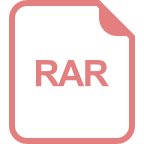
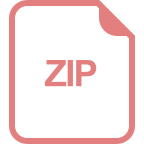
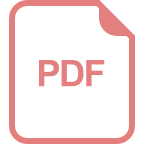
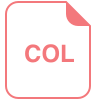
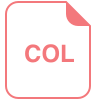
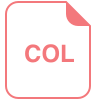
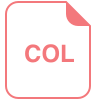
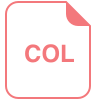
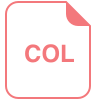







