1、编写一个 PHP 页面,使用 Form 表单上传一个留言,包括作者 ID,时间, 主题,正文,图片。一个留言目前允许上传一个图片,一个留言生成一个文件。 提示,文件的格式可以使用 JSON,便于解析。 2、编写一个页面,对所有的留言进行表格形式的浏览(不显示正文和图片,如 实验二的方式),可以考虑分页的方式(可选,因为要用到 session)。 3、编写一个 php 页面用于显示留言的详细内容,当点击列表中的某个列表时, 用该页面显示详细内容。 4、对展示的表格通过 CSS 或者 HTML 技术进行一定的美化。 5、注意三个页面的导航关系,可以设计一个顶层的导航菜单,或者是其他方式
时间: 2024-01-24 07:17:42 浏览: 90
以下是一个简单的实现方式:
1. 留言上传页面(upload.php)
```php
<!DOCTYPE html>
<html>
<head>
<title>留言上传</title>
</head>
<body>
<h1>留言上传</h1>
<form action="save_message.php" method="post" enctype="multipart/form-data">
<label for="author">作者ID:</label>
<input type="text" name="author" required><br>
<label for="time">时间:</label>
<input type="datetime-local" name="time" required><br>
<label for="subject">主题:</label>
<input type="text" name="subject" required><br>
<label for="content">正文:</label>
<textarea name="content" required></textarea><br>
<label for="image">图片:</label>
<input type="file" name="image"><br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
2. 留言保存页面(save_message.php)
```php
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// 获取表单数据
$author = $_POST["author"];
$time = $_POST["time"];
$subject = $_POST["subject"];
$content = $_POST["content"];
$image = "";
if ($_FILES["image"]["error"] == UPLOAD_ERR_OK) {
// 上传文件
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["image"]["name"]);
move_uploaded_file($_FILES["image"]["tmp_name"], $target_file);
$image = $target_file;
}
// 保存留言到文件
$message = array(
"author" => $author,
"time" => $time,
"subject" => $subject,
"content" => $content,
"image" => $image
);
$messages = array();
if (file_exists("messages.json")) {
// 如果文件存在,读取已有的留言
$messages = json_decode(file_get_contents("messages.json"), true);
}
array_push($messages, $message);
file_put_contents("messages.json", json_encode($messages));
echo "留言已保存。";
}
?>
```
3. 留言列表页面(list_messages.php)
```php
<?php
session_start();
if (!isset($_SESSION["page"])) {
$_SESSION["page"] = 1;
}
$page_size = 10; // 每页显示的留言数
$messages = array();
if (file_exists("messages.json")) {
// 如果文件存在,读取已有的留言
$messages = json_decode(file_get_contents("messages.json"), true);
}
$total_pages = ceil(count($messages) / $page_size); // 总页数
$current_page = $_SESSION["page"];
$start_index = ($current_page - 1) * $page_size; // 当前页第一条留言的索引
$end_index = min($start_index + $page_size, count($messages)); // 当前页最后一条留言的索引(不包含)
?>
<!DOCTYPE html>
<html>
<head>
<title>留言列表</title>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th, td {
text-align: left;
padding: 8px;
border-bottom: 1px solid #ddd;
}
tr:hover {
background-color: #f5f5f5;
}
.pagination {
display: inline-block;
margin-top: 20px;
}
.pagination a {
color: black;
float: left;
padding: 8px 16px;
text-decoration: none;
border: 1px solid #ddd;
}
.pagination a.active {
background-color: #4CAF50;
color: white;
border: 1px solid #4CAF50;
}
.pagination a:hover:not(.active) {
background-color: #ddd;
}
</style>
</head>
<body>
<h1>留言列表</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>时间</th>
<th>主题</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<?php for ($i = $start_index; $i < $end_index; $i++) { ?>
<tr>
<td><?php echo $i + 1 ?></td>
<td><?php echo $messages[$i]["time"] ?></td>
<td><?php echo $messages[$i]["subject"] ?></td>
<td><a href="view_message.php?id=<?php echo $i ?>">查看</a></td>
</tr>
<?php } ?>
</tbody>
</table>
<div class="pagination">
<?php for ($i = 1; $i <= $total_pages; $i++) { ?>
<a href="?page=<?php echo $i ?>"<?php if ($i == $current_page) {echo ' class="active"';} ?>><?php echo $i ?></a>
<?php } ?>
</div>
</body>
</html>
```
4. 留言详情页面(view_message.php)
```php
<?php
if (isset($_GET["id"])) {
$id = $_GET["id"];
$messages = array();
if (file_exists("messages.json")) {
// 如果文件存在,读取已有的留言
$messages = json_decode(file_get_contents("messages.json"), true);
}
if ($id >= 0 && $id < count($messages)) {
$message = $messages[$id];
}
}
?>
<!DOCTYPE html>
<html>
<head>
<title>留言详情</title>
<style>
img {
max-width: 100%;
}
</style>
</head>
<body>
<h1>留言详情</h1>
<?php if (isset($message)) { ?>
<p><strong>作者ID:</strong><?php echo $message["author"] ?></p>
<p><strong>时间:</strong><?php echo $message["time"] ?></p>
<p><strong>主题:</strong><?php echo $message["subject"] ?></p>
<p><strong>正文:</strong><?php echo $message["content"] ?></p>
<?php if (!empty($message["image"])) { ?>
<p><strong>图片:</strong></p>
<img src="<?php echo $message["image"] ?>">
<?php } ?>
<p><a href="list_messages.php">返回列表</a></p>
<?php } else { ?>
<p>留言不存在。</p>
<?php } ?>
</body>
</html>
```
5. 导航菜单(menu.php)
```php
<!DOCTYPE html>
<html>
<head>
<title>留言板</title>
</head>
<body>
<h1>留言板</h1>
<ul>
<li><a href="upload.php">上传留言</a></li>
<li><a href="list_messages.php">浏览留言</a></li>
</ul>
<hr>
</body>
</html>
```
其他页面包含导航菜单:
upload.php:
```php
<?php include("menu.php") ?>
<!-- 留言上传页面内容 -->
```
save_message.php:
```php
<?php include("menu.php") ?>
<!-- 留言保存页面内容 -->
```
list_messages.php:
```php
<?php include("menu.php") ?>
<!-- 留言列表页面内容 -->
```
view_message.php:
```php
<?php include("menu.php") ?>
<!-- 留言详情页面内容 -->
```
注意事项:
1. 上传的文件保存在 uploads 目录下,需要保证该目录有写权限。
2. 为了简化代码,没有进行输入验证和安全处理,请勿直接用于生产环境。
3. 分页使用了 session 来保存当前页码,需要开启 session 功能。
阅读全文
相关推荐
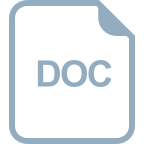
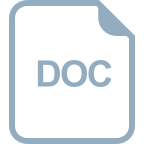
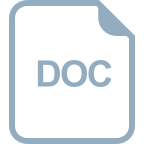















