opencv 如何计算yolov5检测的面积,使用python写出计算yolo检测的面积代码
时间: 2024-03-09 11:44:45 浏览: 168
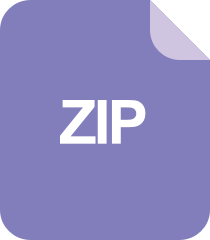
python代码目标检测基于yolov5

要计算YOLOv5检测的面积,需要先检测出物体的边框坐标,然后计算其面积。可以使用OpenCV库中的rectangle函数绘制边框,并使用boundingRect函数获取边框的坐标。然后,可以使用边框的长宽计算面积。下面是一个示例代码:
``` python
import cv2
# 加载YOLOv5模型
net = cv2.dnn.readNet("yolov5.weights", "yolov5.cfg")
# 读取图像
image = cv2.imread("image.jpg")
# 获取图像的高度和宽度
height, width = image.shape[:2]
# 构建一个blob对象,对图像进行预处理
blob = cv2.dnn.blobFromImage(image, 1/255.0, (416, 416), swapRB=True, crop=False)
# 将blob对象输入到模型中进行推理
net.setInput(blob)
# 获取模型的输出
output_layers = net.getUnconnectedOutLayersNames()
outputs = net.forward(output_layers)
# 遍历输出并绘制边框
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > CONFIDENCE_THRESHOLD:
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = int(center_x - w / 2)
y = int(center_y - h / 2)
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 计算面积并输出
area = w * h
print("检测到一个物体,面积为:", area)
# 显示结果
cv2.imshow("YOLOv5检测结果", image)
cv2.waitKey(0)
```
需要注意的是,在这个示例代码中,我们使用了一个名为CONFIDENCE_THRESHOLD的常量来过滤掉低置信度的检测结果。你需要根据自己的实际情况来调整这个值。
阅读全文
相关推荐
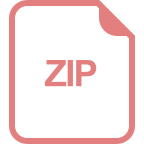
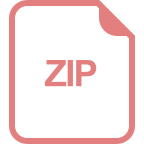
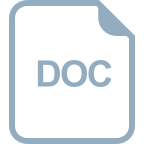
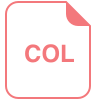
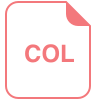
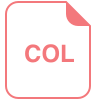
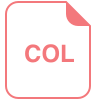









