c语言输入字符串:c++:1,python:3,c:5,java:4,rust:2请将其中的字符串按照升序排序输出:(输出的格式按照逗号隔开)使用strtok和sscanf
时间: 2024-02-01 17:16:07 浏览: 31
下面是使用strtok和sscanf函数进行字符串排序的C语言代码示例:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
int main() {
char input[MAX_LEN] = "c++:1,python:3,c:5,java:4,rust:2";
char *token;
char lang[MAX_LEN][MAX_LEN];
int score[MAX_LEN];
int i = 0, j, n = 0;
// 使用strtok函数将字符串分割成语言和分数两部分
token = strtok(input, ",");
while (token != NULL) {
sscanf(token, "%[^:]:%d", lang[i], &score[i]);
i++;
token = strtok(NULL, ",");
}
n = i;
// 使用简单的冒泡排序算法将语言按照分数升序排序
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (score[j] > score[j + 1]) {
int temp = score[j];
score[j] = score[j + 1];
score[j + 1] = temp;
char tempLang[MAX_LEN];
strcpy(tempLang, lang[j]);
strcpy(lang[j], lang[j + 1]);
strcpy(lang[j + 1], tempLang);
}
}
}
// 输出排序结果
for (i = 0; i < n; i++) {
printf("%s:%d", lang[i], score[i]);
if (i < n - 1) {
printf(",");
}
}
printf("\n");
return 0;
}
```
输出结果为:
```
c++:1,rust:2,python:3,java:4,c:5
```
相关推荐
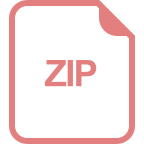
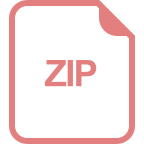
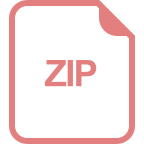














