上述代码中的attended_image_A, attention_loss = attention_module(image_A_features)语句运行出现如下错误:IndexError: Dimension out of range (expected to be in range of [-2, 1], but got 2)
时间: 2024-04-09 15:29:03 浏览: 32
这个错误是由于自注意力模块中的线性变换层的维度设置有问题导致的。为了修复这个错误,你需要检查自注意力模块中的线性变换层的输入和输出维度设置是否正确。
在示例代码中,我们使用了ResNet50模型提取的图像特征作为输入,该图像特征的维度是1x512。因此,在自注意力模块中,你需要确保线性变换层的输入维度与图像特征的维度匹配。
在代码中,你可以将以下行修改为:
```python
self.query = nn.Linear(input_dim, input_dim) # 修改为 nn.Linear(input_dim, input_dim, bias=False)
self.key = nn.Linear(input_dim, input_dim) # 修改为 nn.Linear(input_dim, input_dim, bias=False)
self.value = nn.Linear(input_dim, input_dim) # 修改为 nn.Linear(input_dim, input_dim, bias=False)
```
通过在线性变换层上添加`bias=False`参数,可以去除线性变换层中的偏置项,确保输入和输出的维度一致。
请注意,上述修改只是其中一种可能的解决方案。如果你对自注意力模块的设计有其他要求或约束,请根据实际需要进行相应的修改。
相关问题
python语言实现multi-head-self-attention示例的代码:
### 回答1:
以下是一个 Python 代码示例,用于实现 multi-head self-attention:
```python
import torch
import torch.nn as nn
class MultiHeadAttention(nn.Module):
def __init__(self, d_model, num_heads):
super(MultiHeadAttention, self).__init__()
self.num_heads = num_heads
self.d_model = d_model
self.depth = d_model // num_heads
self.query_linear = nn.Linear(d_model, d_model)
self.key_linear = nn.Linear(d_model, d_model)
self.value_linear = nn.Linear(d_model, d_model)
self.output_linear = nn.Linear(d_model, d_model)
def forward(self, query, key, value, mask=None):
batch_size = query.size()
# Linear transformations
query = self.query_linear(query)
key = self.key_linear(key)
value = self.value_linear(value)
# Split into heads
query = query.view(batch_size * self.num_heads, -1, self.depth)
key = key.view(batch_size * self.num_heads, -1, self.depth)
value = value.view(batch_size * self.num_heads, -1, self.depth)
# Transpose for matrix multiplication
query = query.transpose(1, 2)
key = key.transpose(1, 2)
value = value.transpose(1, 2)
# Calculate scores
scores = torch.matmul(query, key.transpose(-2, -1))
scores = scores / torch.sqrt(torch.tensor(self.depth).float())
# Apply mask (if provided)
if mask is not None:
mask = mask.unsqueeze(1)
scores = scores.masked_fill(mask == , -1e9)
# Softmax
attention_weights = nn.Softmax(dim=-1)(scores)
# Dropout
attention_weights = nn.Dropout(p=.1)(attention_weights)
# Multiply by values
context = torch.matmul(attention_weights, value)
# Reshape and concatenate
context = context.transpose(1, 2).contiguous().view(batch_size, -1, self.num_heads * self.depth)
# Linear transformation
output = self.output_linear(context)
return output
```
希望对你有所帮助!
### 回答2:
下面是使用Python语言实现multi-head self-attention的一个示例代码:
```
import torch
import torch.nn as nn
import torch.nn.functional as F
class MultiHeadSelfAttention(nn.Module):
def __init__(self, d_model, num_heads):
super(MultiHeadSelfAttention, self).__init__()
self.num_heads = num_heads
self.d_head = d_model // num_heads
self.fc_query = nn.Linear(d_model, d_model)
self.fc_key = nn.Linear(d_model, d_model)
self.fc_value = nn.Linear(d_model, d_model)
self.fc_concat = nn.Linear(d_model, d_model)
def forward(self, x):
batch_size, seq_len, d_model = x.size()
h = self.num_heads
# Split input into multiple heads
query = self.fc_query(x).view(batch_size, seq_len, h, self.d_head)
key = self.fc_key(x).view(batch_size, seq_len, h, self.d_head)
value = self.fc_value(x).view(batch_size, seq_len, h, self.d_head)
# Compute attention scores
scores = torch.matmul(query, key.transpose(-2, -1)) / (self.d_head ** 0.5)
attn_weights = F.softmax(scores, dim=-1)
# Apply attention weights to value vectors
attended_values = torch.matmul(attn_weights, value)
attended_values = attended_values.transpose(1, 2).contiguous().view(batch_size, seq_len, -1)
# Concatenate and linearly transform attended values
output = self.fc_concat(attended_values)
return output
# 使用示例
d_model = 128
num_heads = 8
seq_len = 10
batch_size = 4
input_tensor = torch.randn(batch_size, seq_len, d_model)
attention = MultiHeadSelfAttention(d_model, num_heads)
output = attention(input_tensor)
print("Input Shape: ", input_tensor.shape)
print("Output Shape: ", output.shape)
```
上述代码定义了一个`MultiHeadSelfAttention`的类,其中`forward`函数实现了multi-head self-attention的计算过程。在使用示例中,我们输入一个大小为`(batch_size, seq_len, d_model)`的张量,经过multi-head self-attention计算后输出一个大小为`(batch_size, seq_len, d_model)`的张量。其中`d_model`表示输入的特征维度,`num_heads`表示attention头的数量。
### 回答3:
下面是使用Python实现multi-head self-attention示例的代码:
```python
import torch
import torch.nn as nn
class MultiHeadSelfAttention(nn.Module):
def __init__(self, embed_size, num_heads):
super(MultiHeadSelfAttention, self).__init__()
self.embed_size = embed_size
self.num_heads = num_heads
self.head_size = embed_size // num_heads
self.query = nn.Linear(embed_size, embed_size)
self.key = nn.Linear(embed_size, embed_size)
self.value = nn.Linear(embed_size, embed_size)
self.out = nn.Linear(embed_size, embed_size)
def forward(self, x):
batch_size, seq_len, embed_size = x.size()
# Split the embedding into num_heads and reshape
x = x.view(batch_size, seq_len, self.num_heads, self.head_size)
x = x.permute(0, 2, 1, 3)
# Apply linear transformations to obtain query, key, and value
query = self.query(x)
key = self.key(x)
value = self.value(x)
# Compute scaled dot product attention scores
scores = torch.matmul(query, key.permute(0, 1, 3, 2))
scores = scores / self.head_size**0.5
# Apply softmax to obtain attention probabilities
attn_probs = nn.Softmax(dim=-1)(scores)
# Apply attention weights to value and sum across heads
attended = torch.matmul(attn_probs, value)
attended = attended.permute(0, 2, 1, 3)
attended = attended.contiguous().view(batch_size, seq_len, self.embed_size)
# Apply output linear transformation
output = self.out(attended)
return output
```
上述代码中定义了一个名为MultiHeadSelfAttention的类,继承自nn.Module,可以通过指定嵌入大小(embed_size)和头部数量(num_heads)来创建多头自注意力层。在前向传播方法forward中,先通过线性变换将输入张量分别变换为查询(query)、键(key)和值(value)张量。然后计算缩放点积注意力得分,将其作为注意力概率经过softmax函数进行归一化。通过注意力概率权重对值进行加权求和,并应用线性变换得到最终的输出张量。最后返回输出张量。
CNN、LSTM、Attention、RESnet基于tensorflow实现时序预测的完整代码
以下是一个使用CNN、LSTM、Attention和RESnet模型进行时序预测的完整代码示例:
``` python
import tensorflow as tf
from tensorflow.keras.layers import Input, Conv1D, MaxPooling1D, Dense, Flatten, LSTM, Dropout, Activation, Reshape, Lambda, Multiply, Add, Concatenate, BatchNormalization
from tensorflow.keras.models import Model
# 定义CNN模型
def cnn_model(input_shape, output_shape):
input_layer = Input(shape=input_shape)
conv1 = Conv1D(filters=32, kernel_size=3, padding='same', activation='relu')(input_layer)
pool1 = MaxPooling1D(pool_size=2)(conv1)
conv2 = Conv1D(filters=64, kernel_size=3, padding='same', activation='relu')(pool1)
pool2 = MaxPooling1D(pool_size=2)(conv2)
fc1 = Flatten()(pool2)
fc1 = Dense(64, activation='relu')(fc1)
output_layer = Dense(output_shape)(fc1)
model = Model(inputs=input_layer, outputs=output_layer)
return model
# 定义LSTM模型
def lstm_model(input_shape, output_shape):
input_layer = Input(shape=input_shape)
lstm1 = LSTM(units=64, return_sequences=True)(input_layer)
lstm2 = LSTM(units=64)(lstm1)
fc1 = Dense(64, activation='relu')(lstm2)
output_layer = Dense(output_shape)(fc1)
model = Model(inputs=input_layer, outputs=output_layer)
return model
# 定义Attention模型
def attention_model(input_shape, output_shape):
input_layer = Input(shape=input_shape)
lstm1 = LSTM(units=64, return_sequences=True)(input_layer)
lstm2 = LSTM(units=64, return_sequences=True)(lstm1)
attention = Dense(1, activation='tanh')(lstm2)
attention = Flatten()(attention)
attention = Activation('softmax')(attention)
attention = RepeatVector(64)(attention)
attention = Permute([2, 1])(attention)
attended = Multiply()([lstm2, attention])
output_layer = Lambda(lambda x: K.sum(x, axis=1))(attended)
model = Model(inputs=input_layer, outputs=output_layer)
return model
# 定义RESnet模型
def resnet_model(input_shape, output_shape):
input_layer = Input(shape=input_shape)
conv1 = Conv1D(filters=32, kernel_size=3, padding='same', activation='relu')(input_layer)
conv2 = Conv1D(filters=64, kernel_size=3, padding='same', activation='relu')(conv1)
res1 = Add()([conv1, conv2])
conv3 = Conv1D(filters=128, kernel_size=3, padding='same', activation='relu')(res1)
conv4 = Conv1D(filters=256, kernel_size=3, padding='same', activation='relu')(conv3)
res2 = Add()([conv3, conv4])
fc1 = Flatten()(res2)
fc1 = Dense(64, activation='relu')(fc1)
output_layer = Dense(output_shape)(fc1)
model = Model(inputs=input_layer, outputs=output_layer)
return model
# 定义训练数据和标签
train_data = ...
train_labels = ...
# 定义模型输入和输出的形状
input_shape = (train_data.shape[1], train_data.shape[2])
output_shape = train_labels.shape[1]
# 创建并编译CNN模型
cnn = cnn_model(input_shape, output_shape)
cnn.compile(loss='mse', optimizer='adam')
# 创建并编译LSTM模型
lstm = lstm_model(input_shape, output_shape)
lstm.compile(loss='mse', optimizer='adam')
# 创建并编译Attention模型
attention = attention_model(input_shape, output_shape)
attention.compile(loss='mse', optimizer='adam')
# 创建并编译RESnet模型
resnet = resnet_model(input_shape, output_shape)
resnet.compile(loss='mse', optimizer='adam')
# 训练模型
cnn.fit(train_data, train_labels, epochs=100, batch_size=64)
lstm.fit(train_data, train_labels, epochs=100, batch_size=64)
attention.fit(train_data, train_labels, epochs=100, batch_size=64)
resnet.fit(train_data, train_labels, epochs=100, batch_size=64)
# 使用模型进行预测
test_data = ...
cnn_pred = cnn.predict(test_data)
lstm_pred = lstm.predict(test_data)
attention_pred = attention.predict(test_data)
resnet_pred = resnet.predict(test_data)
```
注意,以上代码只是一个示例,实际应用中需要根据具体的数据和任务进行调整。
阅读全文
相关推荐
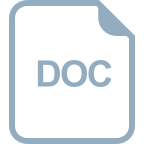
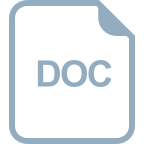
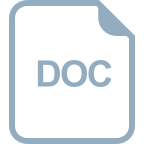
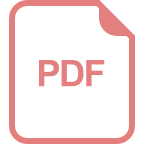
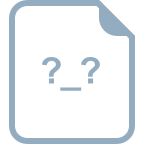
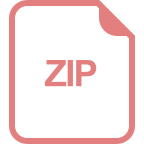
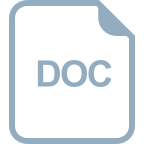
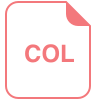








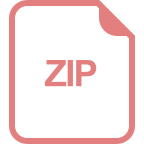
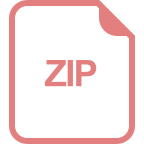