#include <iostream> #include <queue> using namespace std; #define MVNum 50 typedef struct { bool visited[MVNum];//辅助数组 int arcs[MVNum][MVNum];//领接矩阵 int n;// }AMGraph;//领接矩阵的结构体 AMGraph G; queue<int>Q; void BFSTraverse(int v, int n) {//v是输入的数从什么开始遍历 cout << v << " "; G.visited[v] = true; Q.push(v); int front; while (!Q.empty()) { front = Q.front();//输出队头元素 Q.pop();//弹出队头元素 for (int i = 0; i < n; i++) { if (G.arcs[front][i] != 0 && G.visited[i] == false) { cout << i << " "; G.visited[i] = true; Q.push(i); } } } } int main() { cin >> G.n; for (int i = 0; i < G.n; i++) { G.visited[i] = false; for (int j = 0; j < G.n; j++) { scanf("%d", &G.arcs[i][j]); } } BFSTraverse(0, G.n); return 0; }
时间: 2024-01-23 21:02:23 浏览: 133
好的,这是一段 C++ 代码,是一个基于邻接矩阵的图的广度优先遍历算法。
以下是转换后的 Python 代码:
```python
from collections import deque
class AMGraph:
def __init__(self, n):
self.visited = [False] * n
self.arcs = [[0] * n for _ in range(n)]
self.n = n
def BFS_traverse(v, G):
print(v, end=' ')
G.visited[v] = True
Q = deque([v])
while Q:
front = Q.popleft()
for i in range(G.n):
if G.arcs[front][i] != 0 and not G.visited[i]:
print(i, end=' ')
G.visited[i] = True
Q.append(i)
if __name__ == '__main__':
n = int(input())
G = AMGraph(n)
for i in range(n):
for j, x in enumerate(map(int, input().split())):
G.arcs[i][j] = x
BFS_traverse(0, G)
```
注意,Python 的列表下标从 0 开始,所以在 Python 代码中的数组下标要相应地减 1。另外,Python 中没有类似于 C++ 的 `scanf` 函数,可以使用 `map` 函数和 `input` 函数来读取多个整数。
阅读全文
相关推荐
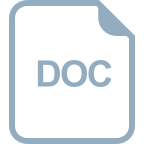
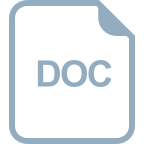
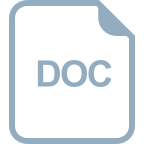






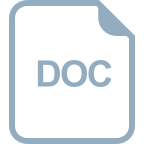
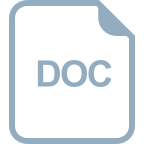
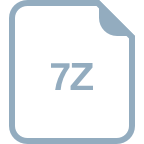
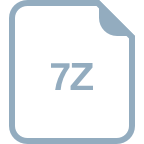