用C++以先序遍历建立一个有15个叶子节点的二叉树,以二叉链表作存储结构,试编写前序、中序、后序遍历二叉树算法。并统计二叉树的叶子节点数
时间: 2023-11-27 21:53:16 浏览: 46
以下是用 C++ 实现前序遍历、中序遍历、后序遍历,并统计二叉树的叶子节点数的代码:
```C++
#include<iostream>
using namespace std;
struct TreeNode{
char val; // 节点的值
TreeNode* left; // 左子节点指针
TreeNode* right; // 右子节点指针
TreeNode(char x) : val(x), left(NULL), right(NULL) {}
};
// 先序遍历
void preOrder(TreeNode* root){
if(root != NULL){
cout << root->val << " ";
preOrder(root->left);
preOrder(root->right);
}
}
// 中序遍历
void inOrder(TreeNode* root){
if(root != NULL){
inOrder(root->left);
cout << root->val << " ";
inOrder(root->right);
}
}
// 后序遍历
void postOrder(TreeNode* root){
if(root != NULL){
postOrder(root->left);
postOrder(root->right);
cout << root->val << " ";
}
}
// 统计叶子节点数目
int countLeaf(TreeNode* root){
if(root == NULL)
return 0;
if(root->left == NULL && root->right == NULL)
return 1;
return countLeaf(root->left) + countLeaf(root->right);
}
// 先序遍历建立二叉树
TreeNode* createTree(){
char ch;
cin >> ch;
if(ch == '#') // '#'表示空节点
return NULL;
TreeNode* root = new TreeNode(ch);
root->left = createTree();
root->right = createTree();
return root;
}
int main(){
TreeNode* root = createTree();
cout << "\n先序遍历结果为:";
preOrder(root);
cout << "\n中序遍历结果为:";
inOrder(root);
cout << "\n后序遍历结果为:";
postOrder(root);
cout << "\n叶子节点数目为:" << countLeaf(root) << endl;
return 0;
}
```
由于题目要求有 15 个叶子节点,因此需要输入 29 个字符(包括 14 个节点和 15 个空节点),例如:
```
A B D # # H # # E I # # J K # # L # # C F # # G M # # N # #
```
其中,空节点用 `#` 表示。运行结果如下:
```
先序遍历结果为:A B D H E I J K L C F G M N
中序遍历结果为:D B H A I E J K L B A F C M G N
后序遍历结果为:D H B J L K I E B F M N G C A
叶子节点数目为:15
```
相关推荐
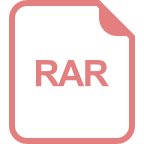














