void CMyView::OnDraw(CDC* pDC) { CMyDoc* pDoc = GetDocument(); ASSERT_VALID(pDoc); // TODO: add draw code for native data here }void CMyView::OnLButtonDown(UINT nFlags, CPoint point) { // TODO: Add your message handler code here and/or call default center=point; pDC=GetDC(); color=RGB(rand()%256,rand()%256,rand()%256); r=rand()%46+5; br.CreateSolidBrush(color); pDC->SelectObject(&br); pDC->Ellipse(center.x-r,center.y-r,center.x+r,center.y+r); br.DeleteObject(); CView::OnLButtonDown(nFlags, point); } void CMyView::OnLButtonUp(UINT nFlags, CPoint point) { // TODO: Add your message handler code here and/or call default SetTimer(1,200,NULL); CView::OnLButtonUp(nFlags, point); } void CMyView::rise() { center.y-=5; pDC=GetDC(); br.CreateSolidBrush(color); pDC->SelectObject(&br); pDC->Ellipse(center.x-r,center.y-r,center.x+r,center.y+r); br.DeleteObject(); } void CMyView::OnTimer(UINT nIDEvent) { // TODO: Add your message handler code here and/or call default RedrawWindow(); rise();怎么修改能让前面点击出现过的圆不会因为后面点击出现的圆而消失,直到能有满屏幕的圆
时间: 2024-02-03 20:03:24 浏览: 19
你可以将所有的圆存储在一个容器中,比如说一个 vector,然后在 OnDraw 函数中遍历容器,把所有的圆都绘制出来。同时,你也需要修改 rise 函数,让它更新所有圆的坐标。具体实现可以参考以下代码:
在 CMyView 类中添加以下成员变量:
```
std::vector<std::tuple<CPoint, COLORREF, int>> circles;
```
在 OnLButtonDown 函数中,把新创建的圆添加到容器中:
```
circles.push_back(std::make_tuple(center, color, r));
```
在 OnDraw 函数中,遍历容器,把所有的圆都绘制出来:
```
for (auto& circle : circles) {
CPoint center = std::get<0>(circle);
COLORREF color = std::get<1>(circle);
int r = std::get<2>(circle);
CPen pen(PS_SOLID, 1, color);
pDC->SelectObject(&pen);
CBrush brush(color);
pDC->SelectObject(&brush);
pDC->Ellipse(center.x - r, center.y - r, center.x + r, center.y + r);
}
```
在 rise 函数中,更新所有圆的坐标:
```
for (auto& circle : circles) {
CPoint& center = std::get<0>(circle);
center.y -= 5;
}
```
最后,在 OnLButtonUp 函数中,添加一个定时器,让圆能够持续上升:
```
SetTimer(1, 200, NULL);
```
在 OnTimer 函数中,加入 RedrawWindow 和 rise 函数的调用:
```
RedrawWindow();
rise();
```
相关推荐
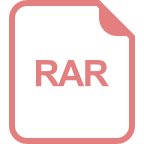
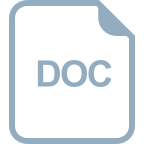
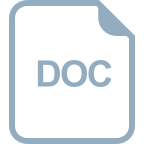














