要求:在下列程序的适当位置添加代码,使用成员函数方法实现下列运算符重载: (1) 复数 + 实数(double类型) 运算; (2) 复数 * 复数 运算。 程序代码: #include <iostream> using namespace std; class Complex { private: double real,image; //分别为实部、虚部 public: Complex(double rel=0, double img=0) { real=rel; image=img; } void display() //输出(a+b*i) 、(a-b*i) 、(a)等形式 { cout<<" ("<<real; if (image>0) cout<<"+"<<image<<"*i) "; //虚部为正 else if (image<0) cout<<image<<"*i) "; //虚部为负 else cout<<") "; //虚部为0,即为实数 } Complex operator +(Complex & c); //用成员函数重载 }; //成员函数的类外定义 Complex Complex::operator +(Complex & c) { Complex temp; temp.real=real+c.real; temp.image=image+c.image; return temp; } int main() { Complex c1(1,2), c2(3,-4),c3,c4; c3=c1+123.456; c4=c1*c2; //输出加的结果 c1.display(); cout<<"+"; cout<<123.456; cout<<"="; c3.display(); cout<<endl; //输出*的结果 c1.display(); cout<<"*"; c2.display(); cout<<"="; c4.display (); cout<<endl; return 0; }
时间: 2024-01-29 21:02:13 浏览: 26
在类中添加以下代码实现运算符重载:
```c++
Complex operator +(double d); //用成员函数重载
Complex operator *(Complex & c); //用成员函数重载
```
在类外定义以下代码实现运算符重载:
```c++
Complex Complex::operator +(double d) {
Complex temp;
temp.real=real+d;
temp.image=image;
return temp;
}
Complex Complex::operator *(Complex & c) {
Complex temp;
temp.real=real*c.real-image*c.image; //实部计算
temp.image=image*c.real+real*c.image; //虚部计算
return temp;
}
```
注意:在成员函数的定义中,不需要再重新声明函数参数的类型,因为已经在类中声明过了。
相关问题
要求:在下列程序的适当位置添加代码,使用友元函数方法实现下列运算符重载: (1) 复数 / 实数(double类型) 运算; (2) 复数 == 复数 运算; (结果:int型,1表示“true”,0表示“false”) (3) 复数 != 复数 运算。(结果:int型,1表示“true”,0表示“false”)
以下是示例代码,实现了复数类 Complex 的运算符重载:
```
#include<iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0): real(r), imag(i) {}
void print() {
cout << "(" << real << "+" << imag << "i)" << endl;
}
friend Complex operator/(const Complex& c, double d); // 友元函数重载除法运算符
friend int operator==(const Complex& c1, const Complex& c2); // 友元函数重载等于运算符
friend int operator!=(const Complex& c1, const Complex& c2); // 友元函数重载不等于运算符
};
Complex operator/(const Complex& c, double d) {
return Complex(c.real / d, c.imag / d);
}
int operator==(const Complex& c1, const Complex& c2) {
return (c1.real == c2.real) && (c1.imag == c2.imag);
}
int operator!=(const Complex& c1, const Complex& c2) {
return !(c1 == c2);
}
int main() {
Complex c1(3, 4);
Complex c2(3, 4);
Complex c3 = c1 / 2;
c1.print();
c2.print();
c3.print();
cout << "c1==c2 ? 结果:" << (c1==c2) << endl;
cout << "c1!=c2 ? 结果:" << (c1!=c2) << endl;
return 0;
}
```
在上面的代码中,我们使用了 friend 关键字来定义了三个友元函数,分别重载了除法运算符、等于运算符和不等于运算符。这些友元函数可以直接访问 Complex 类的私有成员变量,从而实现了运算符的重载。
请编写函数实现并测试运算符重载的方法。 要求: 1、将运算符重载为成员函数,实现复数加实数、复数减实数的功能;
以下是实现运算符重载的代码示例:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 实部
double imag; // 虚部
public:
Complex(double r = 0, double i = 0) {
real = r;
imag = i;
}
Complex operator+(double num) {
Complex res;
res.real = real + num;
res.imag = imag;
return res;
}
Complex operator-(double num) {
Complex res;
res.real = real - num;
res.imag = imag;
return res;
}
void display() {
cout << real << "+" << imag << "i" << endl;
}
};
int main() {
Complex c1(2, 3);
Complex c2 = c1 + 4; // 复数加实数
Complex c3 = c1 - 1.5; // 复数减实数
c1.display();
c2.display();
c3.display();
return 0;
}
```
运算符重载的关键在于重载运算符的函数需要返回一个新的对象,而不是修改原有对象的值。在上述代码中,我们使用了类似于复制构造函数的方式来创建新的对象,并将运算结果存储在该对象中。在 `main` 函数中,我们分别测试了复数加实数和复数减实数的功能。
相关推荐
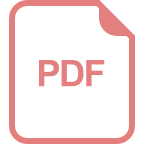










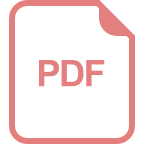
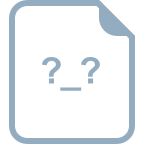