python 函数求导
时间: 2023-10-13 21:21:13 浏览: 168
Python中有一些计算库可以用来求导,比如SymPy和autograd。
使用SymPy进行函数求导可以按照以下步骤:
1. 导入SymPy库
```python
import sympy as sp
```
2. 定义变量和函数
```python
x = sp.Symbol('x')
y = sp.Function('y')(x)
```
3. 对函数进行求导
```python
dy_dx = sp.diff(y, x)
```
4. 打印结果
```python
print(dy_dx)
```
完整的代码示例:
```python
import sympy as sp
# 定义变量和函数
x = sp.Symbol('x')
y = sp.Function('y')(x)
# 对函数进行求导
dy_dx = sp.diff(y, x)
# 打印结果
print(dy_dx)
```
使用autograd进行函数求导可以按照以下步骤:
1. 安装autograd库
```python
!pip install autograd
```
2. 导入autograd库
```python
import autograd.numpy as np
from autograd import grad
```
3. 定义函数
```python
def f(x):
return x**3 + 2*x**2 + 1
```
4. 对函数进行求导
```python
df_dx = grad(f)
```
5. 计算导数值
```python
x = 2
df_dx_value = df_dx(x)
```
6. 打印结果
```python
print(df_dx_value)
```
完整的代码示例:
```python
!pip install autograd
import autograd.numpy as np
from autograd import grad
# 定义函数
def f(x):
return x**3 + 2*x**2 + 1
# 对函数进行求导
df_dx = grad(f)
# 计算导数值
x = 2
df_dx_value = df_dx(x)
# 打印结果
print(df_dx_value)
```
阅读全文
相关推荐
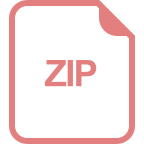
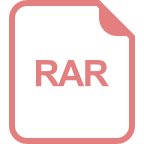
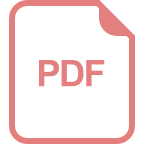















