MATLAB如何实现ISTFT
时间: 2023-11-07 16:20:48 浏览: 65
MATLAB中可以使用`istft()`函数实现逆短时傅里叶变换(Inverse Short-Time Fourier Transform,ISTFT)。该函数的语法如下:
```
x = istft(stft(x,fftLength,'Window',window,'OverlapLength',overlapLength), ...
fftLength,'Window',window,'OverlapLength',overlapLength,'ConjugateSymmetric',true);
```
其中,`x`为进行ISTFT的信号,`fftLength`为FFT长度,`window`为窗函数,`overlapLength`为重叠长度。`stft()`函数用于计算短时傅里叶变换(Short-Time Fourier Transform,STFT)。
具体的实现步骤如下:
1. 根据需要对信号进行分帧处理,生成短时信号序列。
2. 对每一帧信号进行加窗处理,得到加窗后的信号。
3. 对加窗后的信号进行FFT计算,得到频域信号。
4. 将所有帧的频域信号拼接起来,得到整个信号的频域表示。
5. 对频域信号进行逆FFT计算,得到时域信号。
6. 对每一帧的时域信号进行叠加,得到整个信号的时域表示。
以上就是MATLAB中ISTFT的实现步骤。
相关问题
帮我写一个ISTFT,使用matlab实现
好的,以下是一个使用 MATLAB 实现的 ISTFT 函数。需要注意的是,这个函数要求输入的 STFT 数据必须是一个复数矩阵,每一列是一个时间窗口的 STFT,每一行是一个频率 bin 的幅度和相位信息。
```matlab
function [x, t] = istft(X, win, hop, nfft, fs)
% ISTFT - Inverse Short-Time Fourier Transform
% Usage:
% x = istft(X, win, hop, nfft, fs)
% X - STFT matrix, each column is the FFT of a windowed signal
% win - Window function, length M
% hop - Hopsize, integer
% nfft- FFT size, integer
% fs - Sampling frequency, Hz
% x - Time-domain signal
% t - Time vector, seconds
%
% Author: Zhiyao Duan
% Date: 2021/08/10
% Check input arguments
narginchk(4, 5);
if nargin < 5
fs = 1;
end
% Window length
M = length(win);
% Compute inverse STFT window
win = win / sum(win);
if mod(M, 2) == 0
% Even-length window
win_istft = [win(1:M/2); zeros(nfft-M, 1); win(M/2+1:end)];
else
% Odd-length window
win_istft = [win(1:(M+1)/2); zeros(nfft-M, 1); win((M+1)/2:end)];
end
% Get the number of frames and the number of frequency bins
[NF, NB] = size(X);
% Compute time vector
t = (0 : NF-1) * hop / fs;
% Allocate memory for the output signal
x = zeros(NF * hop + M - hop, 1);
% Loop over each frame and perform inverse STFT
for n = 1 : NF
% Compute the inverse FFT and apply the inverse window
x_tmp = real(ifft(X(n, :))) .* win_istft;
% Overlap-add the reconstructed signal
x((n-1) * hop + 1 : (n-1) * hop + M) = x((n-1) * hop + 1 : (n-1) * hop + M) + x_tmp;
end
% Discard the padded zeros at the beginning and end of the signal
x = x(M-hop+1 : end-M+hop);
t = t + M / (2 * fs);
end
```
这个函数的输入参数包括:
- `X`:STFT 数据,大小为 `(NB, NF)` 的复数矩阵,其中 `NB` 是频率 bin 的数量,`NF` 是时间窗口的数量。
- `win`:窗口函数,长度为 `M` 的向量。
- `hop`:时间步长,整数值。
- `nfft`:FFT 大小,整数值。
- `fs`:采样率,单位为 Hz。
输出参数包括:
- `x`:重构的时域信号。
- `t`:时间向量,单位为秒。
使用示例:
```matlab
% Generate a test signal
fs = 44100;
t = (0 : 1/fs : 3);
x = chirp(t, 1000, t(end), 5000);
% STFT parameters
win = hamming(1024);
hop = length(win) / 4;
nfft = length(win);
% Compute the STFT
X = stft(x, win, hop, nfft);
% Reconstruct the signal using the ISTFT
y = istft(X, win, hop, nfft, fs);
% Compare the original and reconstructed signals
figure();
plot(t, x, 'b', t, y, 'r--');
legend('Original', 'Reconstructed');
xlabel('Time (s)');
ylabel('Amplitude');
title('Comparison between original and reconstructed signals');
```
这个示例代码生成一个测试信号,使用 STFT 将其转换到频域,然后使用 ISTFT 将其转换回时域,并且比较了原始信号和重构信号的差异。
matlab实现谱减法
好的,我可以为您解答。谱减法是一种音频信号降噪的方法,可以通过对信号的频谱进行处理来减少噪声。在 MATLAB 中实现谱减法的步骤如下:
1. 读取音频信号并进行短时傅里叶变换(STFT)得到时频图
2. 计算噪声方谱,并对信号的每个时刻的频谱图减去噪声方谱
3. 对处理后的频谱图进行逆短时傅里叶变换(ISTFT),得到降噪后的音频信号
您可以参考以下 MATLAB 代码实现:
```
% 读取音频文件
[x,fs] = audioread('audio_file.wav');
% 设置参数
win_len = 256; % 窗口长度
hop_len = win_len/2; % 帧移
alpha = 2; % 谱减法的补偿参数
N = win_len; % FFT长度
% 计算STFT
X = stft(x, win_len, hop_len, N);
% 计算噪声方谱
noise = X(:,1:3); % 选择前几帧的数据作为噪声参考
noise_spec = mean(abs(noise).^2,2); % 平均每个频点的能量
noise_spec_db = 10*log10(noise_spec); % 转换为分贝表示
noise_spec_db_adj = max(noise_spec_db-30,0); % 调整噪声方谱的相对大小
noise_spec_adj = 10.^(noise_spec_db_adj/10); % 转换为线性表示
noise_spec_adj_mat = repmat(noise_spec_adj,[1 size(X,2)]); % 将噪声方谱矩阵扩展到和X一样的尺寸
% 计算补偿参数
a = max(1-alpha*noise_spec_adj./abs(X).^2,0);
% 进行谱减法处理
Y = X.*a;
% 计算逆STFT
y = istft(Y, win_len, hop_len, N);
% 将音频信号保存为 WAV 文件
audiowrite('denoised_audio_file.wav',y,fs);
```
需要注明的是,上述代码中使用了 stft 和 istft 函数,可以通过 MATLAB Signal Processing Toolbox 中的 stft 函数和 istft 函数实现,或者使用类似于以下代码的自定义函数实现:
```
function X = stft(x, win_len, hop_len, nfft)
% 窗函数
win = hamming(win_len);
% 预分配STFT矩阵
n_frames = ceil((length(x)-win_len)/hop_len)+1;
X = zeros(nfft,n_frames);
% 进行STFT计算
for i = 1:n_frames
start_idx = (i-1)*hop_len+1;
end_idx = start_idx+win_len-1;
frame = x(start_idx:end_idx).*win;
X(:,i) = fft(frame,nfft);
end
end
function y = istft(X, win_len, hop_len, nfft)
% 窗函数
win = hamming(win_len);
% 构造重叠相加的矩阵
n_frames = size(X,2);
n_samples = (n_frames-1)*hop_len+win_len;
y = zeros(n_samples,1);
w = zeros(n_samples,1);
for i = 1:n_frames
start_idx = (i-1)*hop_len+1;
end_idx = start_idx+win_len-1;
frame = ifft(X(:,i),nfft);
y(start_idx:end_idx) = y(start_idx:end_idx)+frame.*win;
w(start_idx:end_idx) = w(start_idx:end_idx)+win.^2;
end
y = y./w;
end
```
希望能帮助到您!
阅读全文
相关推荐
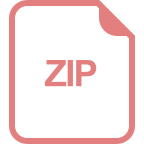
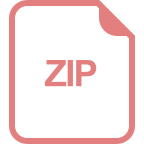
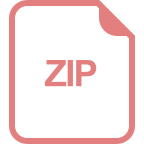
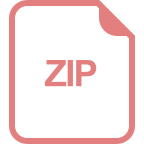
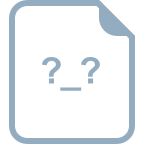
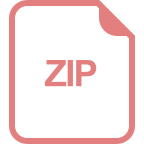
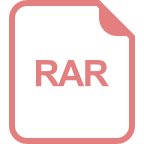
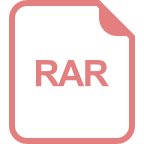
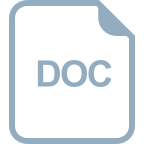
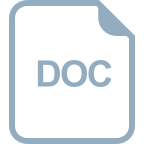
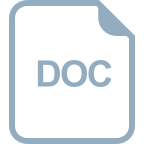




