8个LED循环点亮,利用中断实现当某个按键按下时,LED灯全部闪烁8次,然后再循环点亮。的51程序
时间: 2024-05-08 16:19:55 浏览: 15
以下是一个示例代码,基于STC89C52芯片,实现了8个LED循环点亮以及中断触发的LED闪烁功能。
```c
#include <reg52.h>
// 定义LED端口和按键端口
sbit LED1 = P1^0;
sbit LED2 = P1^1;
sbit LED3 = P1^2;
sbit LED4 = P1^3;
sbit LED5 = P1^4;
sbit LED6 = P1^5;
sbit LED7 = P1^6;
sbit LED8 = P1^7;
sbit KEY = P3^2;
// 定义LED灯闪烁计数器和循环计数器
unsigned char count = 0;
unsigned char cycle = 0;
// 定义函数声明
void initInterrupt();
void initTimer();
void main() {
// 初始化中断和定时器
initInterrupt();
initTimer();
// 初始化LED灯为全灭
LED1 = 1;
LED2 = 1;
LED3 = 1;
LED4 = 1;
LED5 = 1;
LED6 = 1;
LED7 = 1;
LED8 = 1;
while(1) {
// 循环点亮LED灯
LED1 = 0;
delay(100);
LED2 = 0;
delay(100);
LED3 = 0;
delay(100);
LED4 = 0;
delay(100);
LED5 = 0;
delay(100);
LED6 = 0;
delay(100);
LED7 = 0;
delay(100);
LED8 = 0;
delay(100);
LED1 = 1;
LED2 = 1;
LED3 = 1;
LED4 = 1;
LED5 = 1;
LED6 = 1;
LED7 = 1;
LED8 = 1;
// 当按键按下时,LED灯全部闪烁8次
if (count == 8) {
for (cycle = 0; cycle < 8; cycle++) {
LED1 = 0;
LED2 = 0;
LED3 = 0;
LED4 = 0;
LED5 = 0;
LED6 = 0;
LED7 = 0;
LED8 = 0;
delay(100);
LED1 = 1;
LED2 = 1;
LED3 = 1;
LED4 = 1;
LED5 = 1;
LED6 = 1;
LED7 = 1;
LED8 = 1;
delay(100);
}
count = 0;
}
}
}
// 初始化外部中断
void initInterrupt() {
EX0 = 1; // 允许外部中断0
IT0 = 1; // 设置为下降沿触发
}
// 初始化定时器
void initTimer() {
TMOD = 0x01; // 设置定时器0为模式1
TH0 = 0x4C; // 设置定时器初值,每50ms溢出中断一次
TL0 = 0x00;
ET0 = 1; // 允许定时器0中断
TR0 = 1; // 启动定时器0
}
// 中断服务函数
void interruptFunction() interrupt 0 {
if (!KEY) { // 判断按键是否按下
count++; // 计数器加1
}
}
// 定时器中断服务函数,每50ms触发一次
void timerFunction() interrupt 1 {
static unsigned char timer_count = 0;
// 每50ms计数器加1
timer_count++;
if (timer_count == 10) {
timer_count = 0;
}
// 计数器等于5时,触发一次按键检测
if (timer_count == 5) {
if (!KEY) { // 判断按键是否按下
count++; // 计数器加1
}
}
// 重新加载定时器初值,每50ms触发一次
TH0 = 0x4C;
TL0 = 0x00;
}
// 延时函数
void delay(unsigned int time) {
unsigned int i, j;
for (i = time; i > 0; i--) {
for (j = 110; j > 0; j--);
}
}
```
以上代码使用了一个外部中断和一个定时器,实现了按键触发LED闪烁的功能。外部中断检测按键状态,定时器定期检测按键状态。当按键按下时,计数器加1。当计数器等于8时,LED灯全部闪烁8次,并重置计数器。同时,程序还循环点亮8个LED灯。
相关推荐
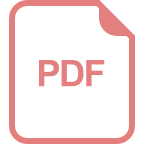














