Create a file writing class Create a new class that writes to the included txt file. Use a BufferedWriter to write the following text to the file: What took you so long? Well, you know, Master, I couldn't find a speeder that I really liked... There he is. ...with an open cockpit and the right speed capabilities. If you spent as much time practicing your saber techniques as you did your wit, you'd rival Master Yoda as a swordsman. I thought I already did. Only in your mind, my very young apprentice. Make sure that all the line breaks are correct. Hint: You can access the file via its relative path by just stating its name: conversation.txt. No need to have the complete path to the file.
时间: 2024-02-13 17:03:00 浏览: 77
Sure, here's an example code for the requested class:
```java
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
public class FileWriterClass {
public static void writeToFile() {
try {
BufferedWriter writer = new BufferedWriter(new FileWriter("conversation.txt"));
writer.write("What took you so long?\n");
writer.write("Well, you know, Master, I couldn't find a speeder that I really liked...\n");
writer.write("There he is.\n");
writer.write("...with an open cockpit and the right speed capabilities.\n");
writer.write("If you spent as much time practicing your saber techniques as you did your wit, you'd rival Master Yoda as a swordsman.\n");
writer.write("I thought I already did.\n");
writer.write("Only in your mind, my very young apprentice.\n");
writer.close();
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
```
This class includes a `writeToFile()` method that creates a `BufferedWriter` object and writes the given text to the specified file name `conversation.txt`. The `BufferedWriter` handles the line breaks automatically, and the code catches and prints any possible IO errors that may occur during the writing process.
You can call this method from anywhere in your code by simply invoking `FileWriterClass.writeToFile()`.
阅读全文
相关推荐
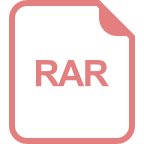












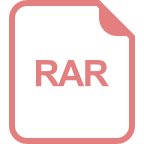
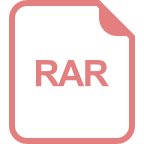
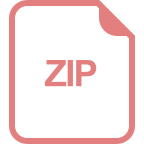
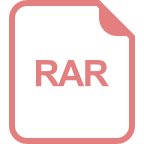