用canvas创建200个随机6种颜色的星星,并且在鼠标移动事件触发时添加30个随机颜色的火花。火花会随机移动,并在逐渐缩小半径后消失。同时,星星也会随机移动,创建出星空的效果。您可以根据需要进行修改和调整。
时间: 2024-02-23 08:03:12 浏览: 75
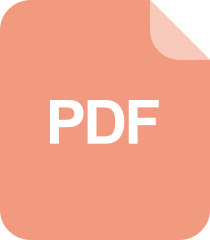
canvas里面如何基于随机点绘制一个多边形的方法
好的,以下是一个示例代码,可以满足您的要求:
HTML代码:
```html
<canvas id="myCanvas"></canvas>
```
JavaScript代码:
```javascript
// 获取canvas元素
const canvas = document.getElementById("myCanvas");
const ctx = canvas.getContext("2d");
// 设置canvas大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 定义星星对象
class Star {
constructor() {
this.x = Math.random() * canvas.width; // 随机x坐标
this.y = Math.random() * canvas.height; // 随机y坐标
this.size = Math.random() * 2; // 随机大小
this.speed = Math.random() * 0.1; // 随机移动速度
this.color = `rgb(${Math.random() * 255}, ${Math.random() * 255}, ${Math.random() * 255})`; // 随机颜色
}
// 绘制星星
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.size, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.fill();
}
// 移动星星
move() {
this.x += this.speed;
if (this.x > canvas.width) {
this.x = 0;
}
}
}
// 定义火花对象
class Spark {
constructor(x, y) {
this.x = x; // 火花的x坐标
this.y = y; // 火花的y坐标
this.radius = 30; // 火花的半径
this.color = `rgb(${Math.random() * 255}, ${Math.random() * 255}, ${Math.random() * 255})`; // 随机颜色
this.vx = Math.random() * 6 - 3; // 随机x方向的速度
this.vy = Math.random() * 6 - 3; // 随机y方向的速度
this.alpha = 1; // 初始透明度
this.fade = Math.random() * 0.05 + 0.02; // 透明度每帧的变化量
}
// 绘制火花
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2);
ctx.fillStyle = this.color;
ctx.globalAlpha = this.alpha;
ctx.fill();
}
// 移动火花
move() {
this.x += this.vx;
this.y += this.vy;
this.radius -= 0.5;
this.alpha -= this.fade;
}
// 判断火花是否消失
isDead() {
return this.radius <= 0 || this.alpha <= 0;
}
}
// 创建200个星星
const stars = [];
for (let i = 0; i < 200; i++) {
stars.push(new Star());
}
// 创建火花数组
const sparks = [];
// 绘制画面
function draw() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 绘制星星
stars.forEach(star => {
star.draw();
star.move();
});
// 绘制火花
sparks.forEach(spark => {
spark.draw();
spark.move();
});
// 移除已经消失的火花
for (let i = sparks.length - 1; i >= 0; i--) {
if (sparks[i].isDead()) {
sparks.splice(i, 1);
}
}
// 每帧重绘
requestAnimationFrame(draw);
}
// 监听鼠标移动事件
canvas.addEventListener("mousemove", event => {
// 添加30个火花
for (let i = 0; i < 30; i++) {
sparks.push(new Spark(event.clientX, event.clientY));
}
});
// 开始绘制画面
draw();
```
上述代码会在 canvas 元素中创建 200 个随机颜色的星星,并在鼠标移动事件触发时添加 30 个随机颜色的火花。火花会随机移动,并在逐渐缩小半径后消失。同时,星星也会随机移动,创建出星空的效果。您可以根据需要进行修改和调整。
阅读全文
相关推荐
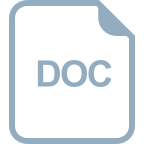
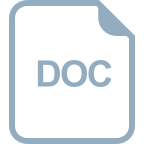
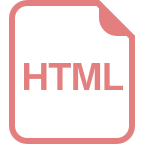
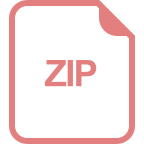
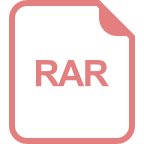
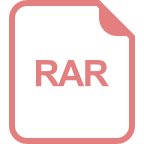
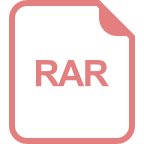
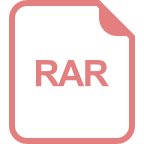
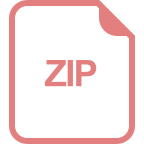
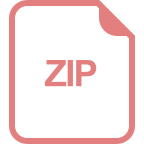
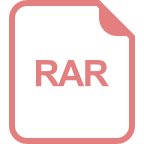
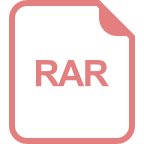
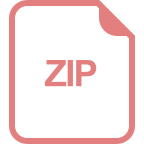
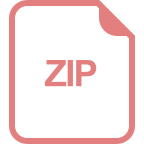
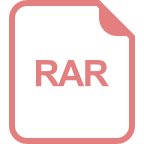