pytest.mark.parametrize与yaml如何使用
时间: 2024-05-02 14:23:57 浏览: 220
pytest.mark.parametrize 和 YAML 都可以用于参数化测试,下面分别介绍它们的使用方法:
1. pytest.mark.parametrize
`pytest.mark.parametrize` 可以用于为测试函数提供参数化输入,其语法如下:
```python
import pytest
@pytest.mark.parametrize("arg1, arg2, expected_output", [
(1, 2, 3),
(10, 20, 30),
(-1, 1, 0),
])
def test_addition(arg1, arg2, expected_output):
assert arg1 + arg2 == expected_output
```
在上面的例子中,`test_addition` 函数被参数化了三次,每次传入的参数分别是 `(1, 2, 3)`,`(10, 20, 30)` 和 `(-1, 1, 0)`。 pytest 会自动运行这个函数三次,并检查每次运行的结果是否符合预期。
2. YAML
YAML 是一种轻量级的数据序列化格式,可以用于存储测试数据。下面是一个使用 YAML 的例子:
```yaml
- name: addition_test
args:
arg1: 1
arg2: 2
expected_output: 3
- name: subtraction_test
args:
arg1: 10
arg2: 5
expected_output: 5
```
在上面的例子中,我们定义了两个测试用例,一个是加法测试,一个是减法测试。每个测试用例包含一个名称和一组参数,这些参数会被传递给测试函数进行测试。
在测试函数中,我们可以使用 PyYAML 库读取 YAML 文件,并将其转换为 Python 对象,然后遍历每个测试用例并运行测试函数。下面是一个示例代码:
```python
import yaml
import pytest
def load_test_data(file_path):
with open(file_path) as f:
test_data = yaml.safe_load(f)
return test_data
def test_addition():
test_data = load_test_data("test_data.yaml")
for test_case in test_data:
args = test_case["args"]
expected_output = args.pop("expected_output")
assert sum(args.values()) == expected_output
def test_subtraction():
test_data = load_test_data("test_data.yaml")
for test_case in test_data:
args = test_case["args"]
expected_output = args.pop("expected_output")
assert args["arg1"] - args["arg2"] == expected_output
```
在上面的代码中,`load_test_data` 函数用于读取 YAML 文件并将其转换为 Python 对象,`test_addition` 和 `test_subtraction` 函数分别用于测试加法和减法。在每个测试函数中,我们先调用 `load_test_data` 函数加载测试数据,然后遍历每个测试用例并运行测试函数。
阅读全文
相关推荐
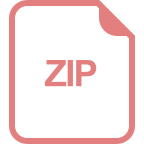
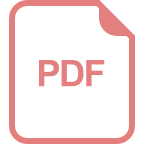









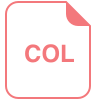
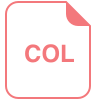
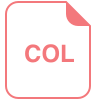
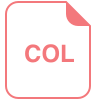
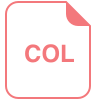
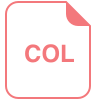
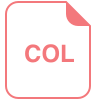