定义一个抽象类 Shape 用以计算面积,从中派生出计算长方形、梯形、圆形面积的派生类。程序中通过基类指针来调用派生类中的虚函数,计算不同形状的面积。
时间: 2023-05-10 19:55:12 浏览: 268
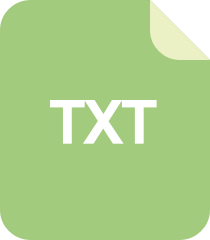
c++例题关于基类与派生类
好的,这是一个经典的面向对象编程问题。以下是一个可能的解决方案:
```c++
#include <iostream>
using namespace std;
class Shape {
public:
virtual double getArea() = 0; // 纯虚函数,用于计算面积
};
class Rectangle : public Shape {
public:
Rectangle(double w, double h) : width(w), height(h) {}
double getArea() { return width * height; }
private:
double width;
double height;
};
class Trapezoid : public Shape {
public:
Trapezoid(double a, double b, double h) : top(a), bottom(b), height(h) {}
double getArea() { return (top + bottom) * height / 2; }
private:
double top;
double bottom;
double height;
};
class Circle : public Shape {
public:
Circle(double r) : radius(r) {}
double getArea() { return 3.1415926 * radius * radius; }
private:
double radius;
};
int main() {
Shape* s1 = new Rectangle(3, 4);
Shape* s2 = new Trapezoid(2, 4, 3);
Shape* s3 = new Circle(5);
cout << "Rectangle area: " << s1->getArea() << endl;
cout << "Trapezoid area: " << s2->getArea() << endl;
cout << "Circle area: " << s3->getArea() << endl;
delete s1;
delete s2;
delete s3;
return 0;
}
```
这个程序定义了一个抽象类 Shape,其中包含一个纯虚函数 getArea(),用于计算面积。然后从 Shape 派生出三个具体的类:Rectangle、Trapezoid 和 Circle,分别用于计算长方形、梯形和圆形的面积。在 main 函数中,我们通过基类指针来调用派生类中的虚函数,计算不同形状的面积。
希望这个解决方案能够帮助你理解如何定义抽象类和派生类,以及如何使用虚函数来实现多态。
阅读全文
相关推荐
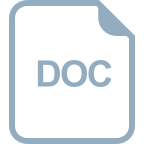
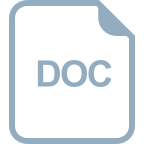


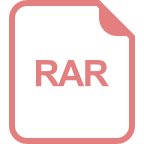



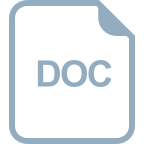
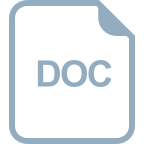
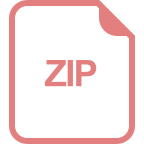
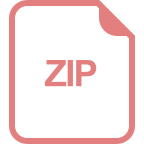
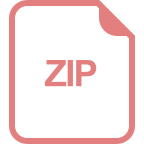
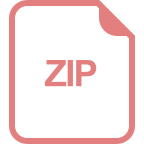
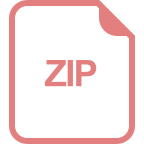
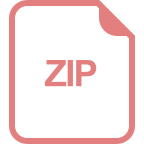
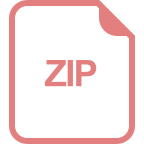