基于D*与动态窗口法的动态路径规划 matlab代码
时间: 2023-07-08 22:43:49 浏览: 38
以下是一个基于D*与动态窗口法的动态路径规划的matlab代码示例:
```matlab
% D*算法路径规划
function [path, cost] = Dstar(start, goal, map)
% 初始化
U = [];
k = 0;
R = Inf(size(map));
R(goal(1), goal(2)) = 0;
U = [U; goal, 0];
% 启发式函数
heuristic = @(pos) norm(pos - goal);
% 移动代价
move_cost = @(pos) map(pos(1), pos(2));
% 动态窗口法
while true
if isempty(U)
path = [];
cost = Inf;
return;
end
% 获取U中距离起点最近的点
[~, idx] = min(U(:,3));
curr = U(idx, :);
if all(curr(1:2) == start)
break;
end
U(idx, :) = [];
k = k + 1;
R(curr(1), curr(2)) = curr(3);
% 获取当前点的邻居节点
neighbors = get_neighbors(curr, map);
for i = 1:size(neighbors, 1)
pos = neighbors(i, :);
if R(pos(1), pos(2)) > R(curr(1), curr(2)) + move_cost(pos) + heuristic(pos) - heuristic(curr(1:2))
R(pos(1), pos(2)) = R(curr(1), curr(2)) + move_cost(pos) + heuristic(pos) - heuristic(curr(1:2));
U = [U; pos, R(pos(1), pos(2)) + heuristic(pos)];
end
end
end
% 生成路径
path = [start; backtrack(start, goal, map, R, heuristic)];
cost = R(start(1), start(2));
end
% 获取当前点的邻居节点
function neighbors = get_neighbors(pos, map)
[row, col] = size(map);
neighbors = [];
if pos(1) > 1
if map(pos(1) - 1, pos(2)) ~= Inf
neighbors = [neighbors; pos(1) - 1, pos(2)];
end
end
if pos(1) < row
if map(pos(1) + 1, pos(2)) ~= Inf
neighbors = [neighbors; pos(1) + 1, pos(2)];
end
end
if pos(2) > 1
if map(pos(1), pos(2) - 1) ~= Inf
neighbors = [neighbors; pos(1), pos(2) - 1];
end
end
if pos(2) < col
if map(pos(1), pos(2) + 1) ~= Inf
neighbors = [neighbors; pos(1), pos(2) + 1];
end
end
end
% 回溯函数
function path = backtrack(start, goal, map, R, heuristic)
path = [goal];
while any(path(1,:) ~= start)
neighbors = get_neighbors(path(1,:), map);
costs = [];
for i = 1:size(neighbors, 1)
pos = neighbors(i,:);
cost = R(pos(1), pos(2)) + norm(pos - path(1,:)) + heuristic(start) - heuristic(pos);
costs = [costs; cost];
end
[~, idx] = min(costs);
path = [neighbors(idx,:); path];
end
end
% 动态路径规划
function [path, cost] = dynamic_path_planning(start, goal, map)
% 初始化
path = [start];
cost = 0;
while true
% 重新规划路径
[new_path, new_cost] = Dstar(path(end,:), goal, map);
% 判断是否无法到达终点
if isempty(new_path) && new_cost == Inf
break;
end
% 判断是否到达终点
if all(new_path(end,:) == goal)
path = [path; new_path(2:end,:)];
cost = cost + new_cost;
break;
end
% 动态调整路径
for i = 2:size(new_path, 1) - 1
if ~is_collision(new_path(i,:), map)
path = [path; new_path(i,:)];
cost = cost + move_cost(path(end-1,:), path(end,:));
else
break;
end
end
end
end
% 判断是否与障碍物发生碰撞
function is_collision = is_collision(pos, map)
is_collision = map(pos(1), pos(2)) == Inf;
end
% 移动代价
function cost = move_cost(pos1, pos2)
cost = norm(pos1 - pos2);
end
```
在使用该代码时,需要传入起点、终点和地图,地图中1表示可通行区域,Inf表示障碍物。其中,Dstar函数实现D*算法路径规划,get_neighbors函数获取当前点的邻居节点,backtrack函数用于回溯生成路径,dynamic_path_planning函数实现动态路径规划,is_collision函数用于判断是否与障碍物发生碰撞,move_cost函数计算移动代价。
相关推荐
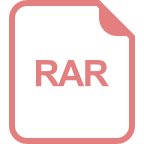
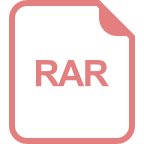
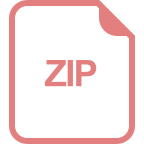














