生成一个C语言数独使之能在dev c++程序中运行
时间: 2024-05-08 16:14:56 浏览: 7
以下是一个简单的C语言数独程序,可以在Dev C++中运行:
```
#include <stdio.h>
#define N 9
#define UNASSIGNED 0
int grid[N][N];
void printGrid() {
for (int row = 0; row < N; row++) {
for (int col = 0; col < N; col++) {
printf("%d ", grid[row][col]);
}
printf("\n");
}
printf("\n");
}
bool findUnassignedLocation(int &row, int &col) {
for (row = 0; row < N; row++) {
for (col = 0; col < N; col++) {
if (grid[row][col] == UNASSIGNED) {
return true;
}
}
}
return false;
}
bool usedInRow(int row, int num) {
for (int col = 0; col < N; col++) {
if (grid[row][col] == num) {
return true;
}
}
return false;
}
bool usedInCol(int col, int num) {
for (int row = 0; row < N; row++) {
if (grid[row][col] == num) {
return true;
}
}
return false;
}
bool usedInBox(int boxStartRow, int boxStartCol, int num) {
for (int row = 0; row < 3; row++) {
for (int col = 0; col < 3; col++) {
if (grid[row+boxStartRow][col+boxStartCol] == num) {
return true;
}
}
}
return false;
}
bool isSafe(int row, int col, int num) {
return !usedInRow(row, num) && !usedInCol(col, num) && !usedInBox(row - row%3, col - col%3, num);
}
bool solveSudoku() {
int row, col;
if (!findUnassignedLocation(row, col)) {
return true;
}
for (int num = 1; num <= 9; num++) {
if (isSafe(row, col, num)) {
grid[row][col] = num;
if (solveSudoku()) {
return true;
}
grid[row][col] = UNASSIGNED;
}
}
return false;
}
int main() {
// Enter the sudoku puzzle here:
int puzzle[N][N] = {
{5, 3, 0, 0, 7, 0, 0, 0, 0},
{6, 0, 0, 1, 9, 5, 0, 0, 0},
{0, 9, 8, 0, 0, 0, 0, 6, 0},
{8, 0, 0, 0, 6, 0, 0, 0, 3},
{4, 0, 0, 8, 0, 3, 0, 0, 1},
{7, 0, 0, 0, 2, 0, 0, 0, 6},
{0, 6, 0, 0, 0, 0, 2, 8, 0},
{0, 0, 0, 4, 1, 9, 0, 0, 5},
{0, 0, 0, 0, 8, 0, 0, 7, 9}
};
// Copy the puzzle into the grid
for (int row = 0; row < N; row++) {
for (int col = 0; col < N; col++) {
grid[row][col] = puzzle[row][col];
}
}
// Solve the puzzle
if (solveSudoku()) {
// Print the solution
printGrid();
} else {
printf("No solution exists\n");
}
return 0;
}
```
将上述代码复制到Dev C++中的一个新项目中,编译并运行该程序即可。
相关推荐














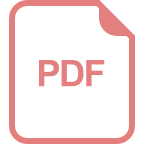