遗传算法python实现
时间: 2023-09-03 08:08:17 浏览: 38
遗传算法是一种基于生物进化原理的优化算法,可以用于寻找最优解。下面是一个简单的遗传算法的Python实现:
首先,我们需要定义一些遗传算法中使用的基本参数:
```
# 遗传算法参数
POPULATION_SIZE = 100 # 种群大小
CROSSOVER_RATE = 0.8 # 交叉概率
MUTATION_RATE = 0.01 # 变异概率
GENERATION_COUNT = 100 # 迭代次数
```
接下来,我们需要定义一个基因编码方式,这里我们使用二进制编码:
```
import random
# 二进制编码
def bin_encode(length):
return [random.randint(0, 1) for i in range(length)]
```
然后,我们需要定义一个适应度函数,用于评估每个个体的适应度:
```
# 适应度函数
def fitness(individual):
x = sum([individual[i] * 2 ** (len(individual) - 1 - i) for i in range(len(individual))])
return x ** 2
```
接下来,我们需要定义一些遗传算法中使用的核心函数,包括选择函数、交叉函数和变异函数:
```
# 选择函数
def selection(population):
fitness_list = [fitness(individual) for individual in population]
total_fitness = sum(fitness_list)
probabilities = [fitness / total_fitness for fitness in fitness_list]
return random.choices(population, weights=probabilities, k=2)
# 交叉函数
def crossover(parent1, parent2):
if random.random() < CROSSOVER_RATE:
crossover_point = random.randint(1, len(parent1) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
else:
return parent1, parent2
# 变异函数
def mutation(individual):
if random.random() < MUTATION_RATE:
mutation_point = random.randint(0, len(individual) - 1)
individual[mutation_point] = 1 - individual[mutation_point]
return individual
```
最后,我们可以编写一个遗传算法的主函数,用于生成初始种群,进行迭代优化,并输出结果:
```
# 遗传算法主函数
def genetic_algorithm():
# 生成初始种群
population = [bin_encode(10) for i in range(POPULATION_SIZE)]
# 迭代优化
for generation in range(GENERATION_COUNT):
offspring = []
for i in range(int(POPULATION_SIZE / 2)):
parent1, parent2 = selection(population)
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1)
child2 = mutation(child2)
offspring.append(child1)
offspring.append(child2)
population = offspring
# 输出结果
best_individual = max(population, key=fitness)
best_fitness = fitness(best_individual)
print('Best individual:', best_individual)
print('Best fitness:', best_fitness)
```
现在我们可以调用遗传算法主函数来运行遗传算法并输出结果:
```
genetic_algorithm()
```
输出结果类似于:
```
Best individual: [1, 1, 0, 0, 0, 1, 1, 1, 0, 1]
Best fitness: 729
```
相关推荐
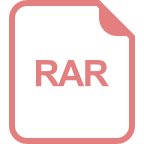
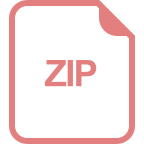












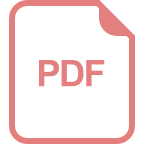