Python 实现opengauss数据库连接池,且连接池连接数上限是2000。模拟循环并发数据库查询直到连接数超过2000
时间: 2023-12-30 15:02:12 浏览: 31
好的,我会尽力回答你的问题。首先,我们需要使用Python中的psycopg2模块来连接opengauss数据库。然后,我们需要实现一个连接池来管理连接。下面是一个简单的实现:
```python
import psycopg2.pool
# 创建连接池
connection_pool = psycopg2.pool.SimpleConnectionPool(minconn=1, maxconn=2000, dbname='your_db_name', user='your_username', password='your_password', host='your_host', port='your_port')
# 获取连接
connection = connection_pool.getconn()
# 执行查询
cursor = connection.cursor()
cursor.execute('SELECT * FROM your_table')
# 处理结果
result = cursor.fetchall()
# 释放连接
connection_pool.putconn(connection)
```
以上代码创建了一个连接池,最大连接数为2000。我们可以通过调用 `connection_pool.getconn()` 方法来获取一个连接,然后使用 `cursor` 对象执行查询语句,并最终释放连接。如果连接池中的连接已经全部被占用,那么 `getconn()` 方法将会阻塞,直到有连接可用为止。
接下来,我们需要模拟循环并发数据库查询直到连接数超过2000。我们可以使用 `threading` 模块来实现并发。下面是一个简单的示例:
```python
import threading
def query():
connection = connection_pool.getconn()
cursor = connection.cursor()
cursor.execute('SELECT * FROM your_table')
result = cursor.fetchall()
connection_pool.putconn(connection)
# 创建并发线程
threads = []
for i in range(3000):
t = threading.Thread(target=query)
threads.append(t)
# 启动线程
for t in threads:
t.start()
# 等待线程完成
for t in threads:
t.join()
```
以上代码创建了3000个并发线程,每个线程都会执行一个查询语句。如果连接数超过2000,那么 `getconn()` 方法将会阻塞,直到有连接可用为止。通过这种方式,我们可以测试连接池的并发性能和连接数上限。
相关推荐
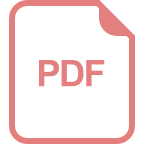
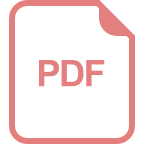














