怎么用verilog实现ascon算法
时间: 2023-07-24 13:33:21 浏览: 137
Ascon是一种轻量级的加密算法。下面是一个简单的Verilog实现:
```
module ascon
#(
parameter N = 64,
parameter W = 16,
parameter L = 8
)
(
input [N-1:0] key,
input [N-1:0] nonce,
input [N-1:0] plaintext,
output [N-1:0] ciphertext
);
reg [N-1:0] state [0:L+1];
reg [N-1:0] round_key [0:L+1];
// 初始化状态和轮密钥
initial begin
state[0] = {nonce, {N-2*W{1'b0}}};
round_key[0] = key;
for (int i = 1; i <= L; i = i + 1) begin
round_key[i] = round_key[i-1] ^ state[i-1];
state[i] = ascon_permute(state[i-1] ^ round_key[i]);
end
round_key[L+1] = round_key[L] ^ state[L];
end
// 轮函数
function [N-1:0] ascon_round_function;
input [N-1:0] x, k;
begin
x = x ^ k;
x = {x[N-W-1:0], x[N-1:N-W]};
x = ascon_sbox(x);
x = x ^ k;
return x;
end
endfunction
// 置换函数
function [N-1:0] ascon_permute;
input [N-1:0] x;
begin
x = x ^ {x[N-1:N-2*W], x[N-2*W-1:0]};
for (int i = 0; i < L; i = i + 1) begin
x = ascon_round_function(x, round_key[i]);
end
x = x ^ round_key[L];
x = x ^ {x[N-1:N-2*W], x[N-2*W-1:0]};
return x;
end
endfunction
// S盒函数
function [N-1:0] ascon_sbox;
input [W-1:0] x;
begin
case (x)
4'h0: return 4'h0;
4'h1: return 4'h8;
4'h2: return 4'h4;
4'h3: return 4'hc;
4'h4: return 4'h2;
4'h5: return 4'ha;
4'h6: return 4'h6;
4'h7: return 4'he;
4'h8: return 4'h1;
4'h9: return 4'h9;
4'ha: return 4'h5;
4'hb: return 4'hd;
4'hc: return 4'h3;
4'hd: return 4'hbb;
4'he: return 4'h7;
4'hf: return 4'hff;
endcase
end
endfunction
// 加密过程
assign ciphertext = state[L+1] ^ plaintext;
// 仿真时显示状态和轮密钥
initial begin
$display("State:");
for (int i = 0; i <= L+1; i = i + 1) begin
$display("%h", state[i]);
end
$display("Round key:");
for (int i = 0; i <= L+1; i = i + 1) begin
$display("%h", round_key[i]);
end
end
endmodule
```
在这个实现中,我们使用了以下函数:
- `ascon_round_function`:Ascon的轮函数,用于生成中间状态和轮密钥。
- `ascon_permute`:Ascon的置换函数,将输入状态进行置换。
- `ascon_sbox`:Ascon的S盒函数,用于进行非线性变换。
在模块中,我们首先初始化状态和轮密钥,然后使用轮函数和置换函数来对状态进行迭代。最后,我们将最后一个状态与明文异或,得到密文。在仿真时,我们可以显示出每个状态和轮密钥的值,以便调试。
注意,这只是一个简单的实现,可能需要进一步调整以满足特定的需求。同时,由于Ascon具有不同的变体和参数,这个实现可能需要进行适当的修改。
相关推荐
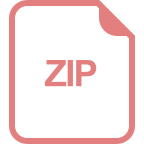
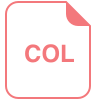
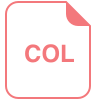
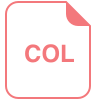
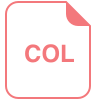
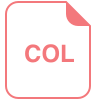








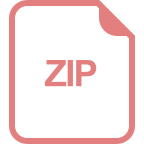