用顺序栈编写程序,依次进栈数据为 1,2,3,4,5,再全部出栈,输出出栈序列。
时间: 2023-08-16 18:08:04 浏览: 65
下面是用 C++ 实现的顺序栈代码:
```cpp
#include <iostream>
using namespace std;
const int MAX_SIZE = 100; // 定义栈的最大容量
class Stack {
private:
int data[MAX_SIZE]; // 存放栈元素的数组
int top; // 栈顶指针
public:
Stack() { top = -1; } // 初始化栈,栈顶指针为 -1
bool isEmpty() { return top == -1; } // 判断栈是否为空
bool isFull() { return top == MAX_SIZE - 1; } // 判断栈是否已满
bool push(int x) { // 将元素 x 进栈
if (isFull()) return false; // 栈已满,无法进栈
data[++top] = x;
return true;
}
bool pop(int& x) { // 将栈顶元素出栈,并将其值存入 x
if (isEmpty()) return false; // 栈为空,无法出栈
x = data[top--];
return true;
}
};
int main() {
Stack s;
int x;
// 依次进栈数据为 1,2,3,4,5
for (int i = 1; i <= 5; ++i) {
s.push(i);
}
// 全部出栈,并输出出栈序列
cout << "出栈序列:";
while (!s.isEmpty()) {
s.pop(x);
cout << x << " ";
}
cout << endl;
return 0;
}
```
输出结果为:
```
出栈序列:5 4 3 2 1
```
相关推荐
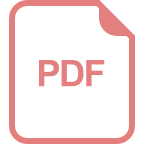









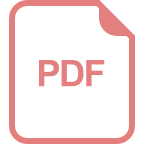
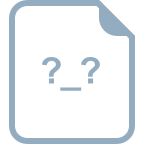