解释这段代码void input(){ srand((unsigned)time(NULL)); int n; string c; cout<<"输入1随机优先级+运行时间(1-5),输入其他字符则自定义参数:"; cin>>c; cout<<"请输入进程数目:"; cin>>n; for(int i=0;i<n;i++){ p=getpch(PCB); if(c=="1"){ cout<<"请输入进程名"<<i+1<<":"; cin>>p->name; p->ntime=rand()%4+1; p->super=rand()%9+1; } else{ cout<<"请分别输入进程名、进程优先数、运行时间:"; cin>>p->name>>p->super>>p->ntime; } p->state='W'; p->rtime=0; sort(); } }
时间: 2024-02-29 07:53:43 浏览: 50
这段代码是一个简单的进程输入函数,主要功能是创建进程并将它们加入就绪队列中。具体地,它首先使用当前时间作为随机数生成器的种子,然后要求用户输入进程个数和进程的属性。如果用户输入的是1,则随机产生每个进程的优先级和运行时间。否则,它将要求用户输入每个进程的名称、优先级和运行时间。接下来,它会为每个进程分配内存,并设置进程的状态为等待状态。然后它调用 sort() 函数将新创建的进程加入就绪队列中。最后,输入函数结束。
相关问题
int main() { // insert code here... clock_t start_time=clock(); srand(unsigned(time(NULL))); int A[100]; const int min=1; const int max=100; cout<<"The previous numbers are:"<<endl; for(int i=0;i<100;i++)A[i]=rand()%(max-min+1)+min; for(int i=0;i<100;i++)cout<<A[i]<<"\t"; cout<<endl; cout<<"The current numbers are:"<<endl; MergeSort(A,100); for(int i=0;i<100;i++)cout<<A[i]<<"\t"; cout<<endl; cout<<"The elapsed time is: "<<double(clock()-start_time)<<"s"<<endl; cout<<endl; return 0; }
这段代码是一个使用归并排序对一组随机数进行排序,并输出排序前后的结果及程序运行时间的程序。
其中,`srand(unsigned(time(NULL)))`是为了使得每次程序运行时生成的随机数序列都不同。
`MergeSort`是一个自定义的归并排序函数,用于对数组进行排序。
程序的输出结果包括排序前的100个随机数,排序后的100个数,以及程序运行时间。
#include <stdlib.h> #include <time.h> #include <iostream.h> int N = 10; void BubbleSort(int a,int n); int main() { srand((unsigned int)time(0)); int a[N]={0}; int i; for(i=0;i<N;i++) a[i] = rand()%100; cout << "The Data Befor Sort:"<< endl; for(i=0;i<N;i++) cout << a[i] << "\t"; cout << endl; BubbleSort(a[N],N); cout << "The Data After Sort:"<< endl; for(i=0;i<=N;i++) cout << a[i] << "\t"; cout << endl; return 0; } void BubbleSort(int a,int n) { int i,k,t; int flag=0; i=0; while(i<n-1) { k = n-1; while(k>i) { if(a[k-1]>a[k]) { t = a[k-1]; a[k-1] = a[k]; a[k] = t; flag = 1; } k--; } i++; if(flag = 0) break; else flag = 0; } }
这段代码中有几个问题:
1. 头文件 `iostream.h` 不应该有 `.h` 后缀,应该写成 `#include <iostream>`。
2. 在 `BubbleSort` 函数中,参数 `int a` 应该写成 `int a[]` 或 `int *a`,因为传入的是数组,而不是单个整数。
3. 在 `main` 函数中,调用 `BubbleSort` 函数时,应该传入数组 `a` 而不是 `a[N]`。
4. 在 `main` 函数中,第二个循环中的条件应该是 `i < N`,而不是 `i <= N`,因为数组下标从 0 开始计数,所以 `a[N]` 是越界的。
下面是修改后的代码:
```
#include <stdlib.h>
#include <time.h>
#include <iostream>
using namespace std;
const int N = 10;
void BubbleSort(int a[], int n);
int main() {
srand((unsigned int)time(0));
int a[N] = {0};
int i;
for (i = 0; i < N; i++)
a[i] = rand() % 100;
cout << "The Data Before Sort:" << endl;
for (i = 0; i < N; i++)
cout << a[i] << "\t";
cout << endl;
BubbleSort(a, N);
cout << "The Data After Sort:" << endl;
for (i = 0; i < N; i++)
cout << a[i] << "\t";
cout << endl;
return 0;
}
void BubbleSort(int a[], int n) {
int i, k, t;
int flag = 0;
i = 0;
while (i < n - 1) {
k = n - 1;
while (k > i) {
if (a[k - 1] > a[k]) {
t = a[k - 1];
a[k - 1] = a[k];
a[k] = t;
flag = 1;
}
k--;
}
i++;
if (flag == 0)
break;
else
flag = 0;
}
}
```
阅读全文
相关推荐
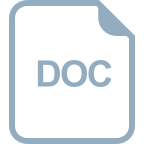
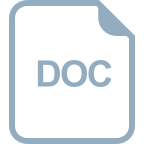
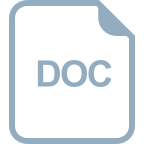













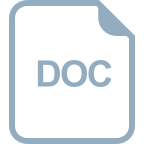