df.iterrows()怎么使用
时间: 2023-04-07 20:01:44 浏览: 166
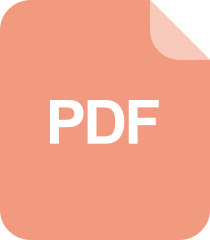
python中使用iterrows()对dataframe进行遍历的实例
df.iterrows()是pandas库中的一个函数,用于遍历DataFrame中的每一行数据。使用方法如下:
```python
import pandas as pd
# 创建一个DataFrame
df = pd.DataFrame({'name': ['Alice', 'Bob', 'Charlie'], 'age': [25, 30, 35]})
# 遍历每一行数据
for index, row in df.iterrows():
print(index, row['name'], row['age'])
```
输出结果为:
```
0 Alice 25
1 Bob 30
2 Charlie 35
```
其中,index表示行的索引,row是一个Series对象,包含了该行的所有数据。可以通过row['列名']来获取该行某一列的数据。
阅读全文
相关推荐
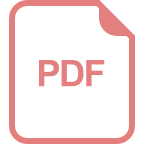
















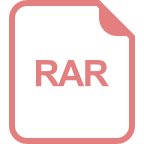