基于顺序存储结构的图书信息表的创建和输出 c语言代码
时间: 2023-04-07 08:02:50 浏览: 102
我可以回答这个问题。基于顺序存储结构的图书信息表可以使用数组来实现。以下是一个简单的示例代码:
#include <stdio.h>
#include <string.h>
#define MAX_BOOKS 100 // 最大图书数量
struct Book {
char title[50]; // 书名
char author[50]; // 作者
int year; // 出版年份
};
struct Book books[MAX_BOOKS]; // 图书信息数组
int num_books = 0; // 当前图书数量
// 添加一本图书
void add_book(char *title, char *author, int year) {
if (num_books >= MAX_BOOKS) {
printf("Error: too many books\n");
return;
}
struct Book book;
strcpy(book.title, title);
strcpy(book.author, author);
book.year = year;
books[num_books++] = book;
}
// 输出所有图书信息
void print_books() {
printf("Title\tAuthor\tYear\n");
for (int i = 0; i < num_books; i++) {
printf("%s\t%s\t%d\n", books[i].title, books[i].author, books[i].year);
}
}
int main() {
add_book("The Catcher in the Rye", "J.D. Salinger", 1951);
add_book("To Kill a Mockingbird", "Harper Lee", 1960);
add_book("1984", "George Orwell", 1949);
print_books();
return 0;
}
相关推荐
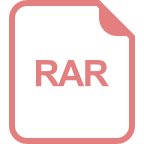














