用c语言创建无向带权图,要求:其结点是字符型
时间: 2024-01-21 17:19:22 浏览: 65
要创建一个无向带权图,需要定义一个结构体来表示图中的节点。在这个结构体中,我们需要定义节点的名称(字符型)和与其相邻节点之间的边的权重(整型)。
以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_NODES 100
typedef struct {
char name;
int weight[MAX_NODES];
} Node;
int main() {
Node nodes[MAX_NODES];
int num_nodes, num_edges;
printf("Enter the number of nodes: ");
scanf("%d", &num_nodes);
for (int i = 0; i < num_nodes; i++) {
printf("Enter the name of node %d: ", i + 1);
scanf(" %c", &(nodes[i].name));
for (int j = 0; j < num_nodes; j++) {
nodes[i].weight[j] = 0;
}
}
printf("Enter the number of edges: ");
scanf("%d", &num_edges);
for (int i = 0; i < num_edges; i++) {
char node1_name, node2_name;
int weight;
printf("Enter the names of the nodes connected by edge %d: ", i + 1);
scanf(" %c %c", &node1_name, &node2_name);
int node1_index = -1;
int node2_index = -1;
for (int j = 0; j < num_nodes; j++) {
if (nodes[j].name == node1_name) {
node1_index = j;
}
if (nodes[j].name == node2_name) {
node2_index = j;
}
}
if (node1_index == -1 || node2_index == -1) {
printf("Error: one or more nodes not found\n");
exit(1);
}
printf("Enter the weight of the edge: ");
scanf("%d", &weight);
nodes[node1_index].weight[node2_index] = weight;
nodes[node2_index].weight[node1_index] = weight;
}
// Output the adjacency matrix
printf("Adjacency matrix:\n");
printf(" ");
for (int i = 0; i < num_nodes; i++) {
printf("%c ", nodes[i].name);
}
printf("\n");
for (int i = 0; i < num_nodes; i++) {
printf("%c ", nodes[i].name);
for (int j = 0; j < num_nodes; j++) {
printf("%d ", nodes[i].weight[j]);
}
printf("\n");
}
return 0;
}
```
这个程序首先提示用户输入节点的数量和边的数量,然后分别提示用户输入每个节点的名称和每个边的两个节点名称和权重。程序将这些信息存储在一个节点数组中,并使用邻接矩阵来表示图的结构。最后,程序输出邻接矩阵以显示图的结构。
阅读全文
相关推荐
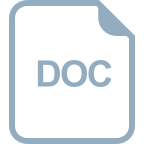
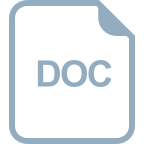
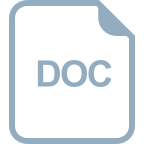



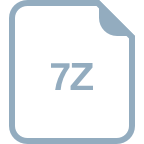
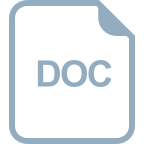
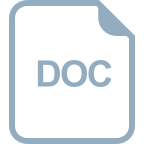
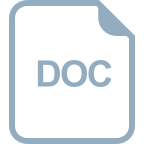
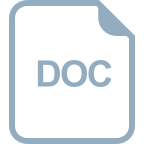
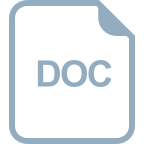
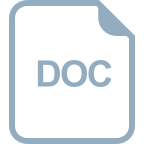

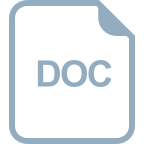
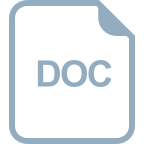
