请你用javascript实现三个 API append,addAll 和 multAll 来实现奇妙序列。 请实现 Fancy 类 : Fancy() 初始化一个空序列对象。 void append(val) 将整数 val 添加在序列末尾。 void addAll(inc) 将所有序列中的现有数值都增加 inc 。 void multAll(m) 将序列中的所有现有数值都乘以整数 m 。 int getIndex(idx) 得到下标为 idx 处的数值(下标从 0 开始),并将结果对 109 + 7 取余。如果下标大于等于序列的长度,请返回 -1 。
时间: 2023-02-06 15:57:07 浏览: 75
以下是用 JavaScript 实现的 Fancy 类的示例代码:
```
class Fancy {
constructor() {
this.sequence = [];
this.increment = 0;
this.multiplier = 1;
}
append(val) {
this.sequence.push(val);
}
addAll(inc) {
this.increment += inc;
}
multAll(m) {
this.multiplier *= m;
this.increment *= m;
}
getIndex(idx) {
if (idx >= this.sequence.length) return -1;
return ((this.sequence[idx] + this.increment) * this.multiplier) % 1000000007;
}
}
```
这个类定义了三个方法:
- `append` 方法用于向序列末尾添加一个整数。
- `addAll` 方法用于将所有序列中的现有数值都增加给定的数。
- `multAll` 方法用于将所有序列中的现有数值都乘以给定的数。
- `getIndex` 方法用于获取序列中下标为 idx 的数值,并将结果对 1000000007 取模。如果下标大于等于序列的长度,则返回 -1。
示例:
```
const fancy = new Fancy();
fancy.append(1);
fancy.append(2);
fancy.append(3);
fancy.addAll(1);
fancy.multAll(2);
console.log(fancy.getIndex(0)); // 输出 4
console.log(fancy.getIndex(1)); // 输出 6
console.log(fancy.getIndex(2)); // 输出 8
console.log(fancy.getIndex(3)); // 输出 -1
```
相关推荐
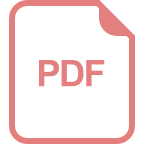
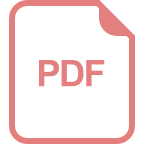
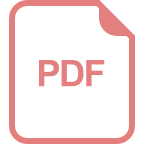














