ros导航机器人模型代码
时间: 2023-12-24 09:05:25 浏览: 58
以下是一个简单的ROS导航机器人模型代码,可以作为参考:
```python
#!/usr/bin/env python
import rospy
from geometry_msgs.msg import Twist
from nav_msgs.msg import Odometry
from sensor_msgs.msg import LaserScan
import tf
import math
class Robot:
def __init__(self):
rospy.init_node('robot_navigation')
self.odom_sub = rospy.Subscriber('/odom', Odometry, self.odom_callback)
self.scan_sub = rospy.Subscriber('/scan', LaserScan, self.scan_callback)
self.cmd_vel_pub = rospy.Publisher('/cmd_vel', Twist, queue_size=10)
self.tf_listener = tf.TransformListener()
self.rate = rospy.Rate(10)
self.cmd_vel = Twist()
self.goal_x = 2.0
self.goal_y = 2.0
self.goal_tolerance = 0.1
self.distance_tolerance = 0.5
self.angle_tolerance = 0.1
def odom_callback(self, msg):
try:
(trans, rot) = self.tf_listener.lookupTransform('/map', '/base_link', rospy.Time(0))
except (tf.LookupException, tf.ConnectivityException, tf.ExtrapolationException):
return
current_x = trans[0]
current_y = trans[1]
current_yaw = tf.transformations.euler_from_quaternion(rot)[2]
distance_to_goal = math.sqrt((self.goal_x - current_x)**2 + (self.goal_y - current_y)**2)
if distance_to_goal < self.goal_tolerance:
self.cmd_vel.linear.x = 0.0
self.cmd_vel.angular.z = 0.0
self.cmd_vel_pub.publish(self.cmd_vel)
rospy.loginfo('Goal reached!')
rospy.signal_shutdown('Goal reached!')
else:
angle_to_goal = math.atan2(self.goal_y - current_y, self.goal_x - current_x)
angle_difference = angle_to_goal - current_yaw
if angle_difference > math.pi:
angle_difference -= 2 * math.pi
elif angle_difference < -math.pi:
angle_difference += 2 * math.pi
if abs(angle_difference) < self.angle_tolerance:
self.cmd_vel.linear.x = 0.5
else:
self.cmd_vel.linear.x = 0.0
if distance_to_goal > self.distance_tolerance:
if angle_difference > self.angle_tolerance:
self.cmd_vel.angular.z = 0.5
elif angle_difference < -self.angle_tolerance:
self.cmd_vel.angular.z = -0.5
else:
self.cmd_vel.angular.z = 0.0
self.cmd_vel_pub.publish(self.cmd_vel)
def scan_callback(self, msg):
# Add obstacle detection and avoidance here
pass
def run(self):
while not rospy.is_shutdown():
self.rate.sleep()
if __name__ == '__main__':
robot = Robot()
robot.run()
```
这个机器人模型会订阅`/odom`和`/scan`话题,发布基于里程计和激光雷达数据的速度控制命令到`/cmd_vel`话题,以达到机器人导航到目标点的目的。你可以根据自己的需求进行修改和扩展。
相关推荐
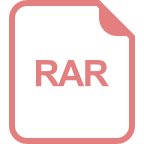
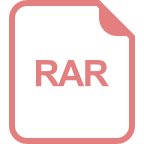














