基于MSP430单片机DS1302的实时时钟设计代码(不基于其它库)
时间: 2024-05-13 16:19:43 浏览: 136
以下是基于MSP430单片机DS1302的实时时钟设计代码,不基于其他库。
```c
#include <msp430g2553.h>
#define DS1302_SCLK BIT2 // P1.2
#define DS1302_IO BIT3 // P1.3
#define DS1302_RST BIT4 // P1.4
// DS1302初始化
void DS1302_Init(void)
{
P1DIR |= DS1302_SCLK + DS1302_IO + DS1302_RST; // 设置IO口方向为输出
P1OUT &= ~(DS1302_SCLK + DS1302_IO + DS1302_RST); // 初始化IO口状态
}
// 向DS1302写入一个字节
void DS1302_WriteByte(unsigned char dat)
{
unsigned char i;
for (i = 0; i < 8; i++)
{
if (dat & 0x01)
P1OUT |= DS1302_IO;
else
P1OUT &= ~DS1302_IO;
P1OUT |= DS1302_SCLK;
P1OUT &= ~DS1302_SCLK;
dat >>= 1;
}
}
// 从DS1302读取一个字节
unsigned char DS1302_ReadByte(void)
{
unsigned char i, dat = 0;
for (i = 0; i < 8; i++)
{
if (P1IN & DS1302_IO)
dat |= (0x01 << i);
P1OUT |= DS1302_SCLK;
P1OUT &= ~DS1302_SCLK;
}
return dat;
}
// 向DS1302写入一个字节的指定位
void DS1302_WriteByteWithBit(unsigned char dat, unsigned char bit)
{
unsigned char temp;
temp = DS1302_ReadByte();
if (dat)
temp |= (0x01 << bit);
else
temp &= ~(0x01 << bit);
DS1302_WriteByte(temp);
}
// 设置DS1302时间
void DS1302_SetTime(unsigned char year, unsigned char month, unsigned char day,
unsigned char hour, unsigned char minute, unsigned char second)
{
DS1302_WriteByteWithBit(0, 7); // 写保护禁止
DS1302_WriteByte(0x80); // 启动充电功能
DS1302_WriteByteWithBit(second / 10, 4);
DS1302_WriteByteWithBit(second % 10, 0);
DS1302_WriteByteWithBit(minute / 10, 4);
DS1302_WriteByteWithBit(minute % 10, 0);
DS1302_WriteByteWithBit(hour / 10, 4);
DS1302_WriteByteWithBit(hour % 10, 0);
DS1302_WriteByteWithBit(day / 10, 4);
DS1302_WriteByteWithBit(day % 10, 0);
DS1302_WriteByteWithBit(month / 10, 4);
DS1302_WriteByteWithBit(month % 10, 0);
DS1302_WriteByteWithBit(year / 10, 4);
DS1302_WriteByteWithBit(year % 10, 0);
DS1302_WriteByteWithBit(0, 7); // 写保护使能
}
// 获取DS1302时间
void DS1302_GetTime(unsigned char *year, unsigned char *month, unsigned char *day,
unsigned char *hour, unsigned char *minute, unsigned char *second)
{
unsigned char temp;
temp = DS1302_ReadByte();
*second = (temp >> 4) * 10 + (temp & 0x0f);
temp = DS1302_ReadByte();
*minute = (temp >> 4) * 10 + (temp & 0x0f);
temp = DS1302_ReadByte();
*hour = (temp >> 4) * 10 + (temp & 0x0f);
temp = DS1302_ReadByte();
*day = (temp >> 4) * 10 + (temp & 0x0f);
temp = DS1302_ReadByte();
*month = (temp >> 4) * 10 + (temp & 0x0f);
temp = DS1302_ReadByte();
*year = (temp >> 4) * 10 + (temp & 0x0f);
}
```
代码解释:
- `DS1302_Init()` : 初始化DS1302,将IO口设置为输出并初始化IO口状态。
- `DS1302_WriteByte()` : 向DS1302写入一个字节。
- `DS1302_ReadByte()` : 从DS1302读取一个字节。
- `DS1302_WriteByteWithBit()` : 向DS1302写入一个字节的指定位。
- `DS1302_SetTime()` : 设置DS1302的时间。
- `DS1302_GetTime()` : 获取DS1302的时间。
要使用这些函数,需要先调用`DS1302_Init()`进行初始化。然后可以使用`DS1302_SetTime()`设置时间,使用`DS1302_GetTime()`获取时间。注意,DS1302的时间是BCD码格式,需要进行转换才能将其显示出来。
阅读全文
相关推荐
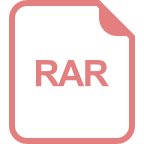
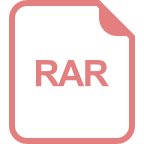
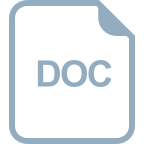
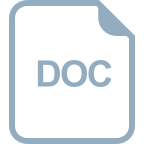

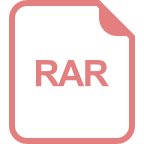
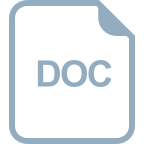
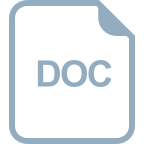
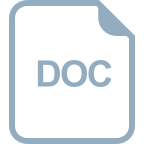



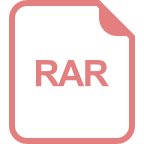
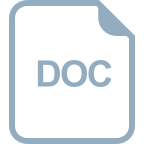
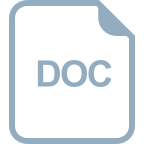
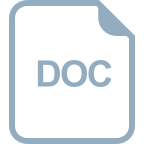

