msp430F149实时时钟设计(1)可使用MSP430单片机内部定时器或实时时钟芯片DS1302;(2)基本显示:年、月、日、时、分、秒(3)可调整时间,设置闹钟 写一个基于MSP430F49芯片使用IAR Embedded Workbench IDE编译器写一个程序
时间: 2024-05-10 10:17:48 浏览: 22
以下是一个基于MSP430F149芯片使用IAR Embedded Workbench IDE编译器的实时时钟设计程序,使用实时时钟芯片DS1302。
```c
#include <msp430.h>
#include "ds1302.h"
#define LED1 BIT0
#define LED2 BIT6
void main(void) {
WDTCTL = WDTPW + WDTHOLD; // 停用看门狗定时器
P1DIR |= LED1 | LED2; // 设置LED1和LED2的I/O方向为输出
ds1302_init(); // 初始化DS1302实时时钟芯片
while (1) {
ds1302_get_time(); // 获取当前时间
P1OUT ^= LED1; // 翻转LED1
if (ds1302_get_seconds() == 0) {
// 如果当前秒数为0,则表示已经进入下一分钟
P1OUT ^= LED2; // 翻转LED2
// 在此处添加闹钟检测代码
}
__delay_cycles(1000000); // 延时1秒
}
}
```
在上述代码中,我们使用`ds1302.h`头文件中的函数来访问DS1302实时时钟芯片。该文件的内容如下:
```c
#ifndef DS1302_H_
#define DS1302_H_
void ds1302_init(void);
void ds1302_get_time(void);
void ds1302_set_time(unsigned char year, unsigned char month, unsigned char day, unsigned char hour, unsigned char minute, unsigned char second);
unsigned char ds1302_get_seconds(void);
unsigned char ds1302_get_minutes(void);
unsigned char ds1302_get_hours(void);
unsigned char ds1302_get_day_of_week(void);
unsigned char ds1302_get_day_of_month(void);
unsigned char ds1302_get_month(void);
unsigned char ds1302_get_year(void);
#endif /* DS1302_H_ */
```
该头文件中包含了多个函数,用于初始化DS1302实时时钟芯片、获取当前时间、设置时间、获取秒数、获取分钟、获取小时、获取星期几、获取日期、获取月份和获取年份等操作。
下面是`ds1302.c`文件的代码,该文件实现了上述头文件中定义的函数。
```c
#include <msp430.h>
#include "ds1302.h"
#define DS1302_SCLK BIT5 // P1.5
#define DS1302_IO BIT6 // P1.6
#define DS1302_CE BIT7 // P1.7
void ds1302_write_byte(unsigned char cmd) {
unsigned char i;
for (i = 0; i < 8; i++) {
if (cmd & 0x01) {
P1OUT |= DS1302_IO;
} else {
P1OUT &= ~DS1302_IO;
}
cmd >>= 1;
P1OUT |= DS1302_SCLK;
P1OUT &= ~DS1302_SCLK;
}
}
unsigned char ds1302_read_byte(void) {
unsigned char i, cmd = 0;
for (i = 0; i < 8; i++) {
cmd >>= 1;
if (P1IN & DS1302_IO) {
cmd |= 0x80;
}
P1OUT |= DS1302_SCLK;
P1OUT &= ~DS1302_SCLK;
}
return cmd;
}
void ds1302_init(void) {
P1DIR |= DS1302_SCLK | DS1302_IO | DS1302_CE; // 将DS1302的SCLK、IO和CE引脚设置为输出
P1OUT &= ~(DS1302_SCLK | DS1302_IO | DS1302_CE); // 将DS1302的SCLK、IO和CE引脚输出低电平
ds1302_write_byte(0x8e); // 写入控制字节
ds1302_write_byte(0); // 禁止写保护
ds1302_set_time(21, 9, 30, 16, 0, 0); // 设置默认时间为2021年9月30日16时0分0秒
}
void ds1302_set_time(unsigned char year, unsigned char month, unsigned char day, unsigned char hour, unsigned char minute, unsigned char second) {
ds1302_write_byte(0xbe); // 写入开始写入时间的命令字节
ds1302_write_byte(second); // 写入秒数
ds1302_write_byte(minute); // 写入分钟
ds1302_write_byte(hour); // 写入小时
ds1302_write_byte(day); // 写入日期
ds1302_write_byte(month); // 写入月份
ds1302_write_byte(year); // 写入年份
ds1302_write_byte(0); // 写入星期几,此处不使用
ds1302_write_byte(0); // 写入保留字节,此处不使用
}
void ds1302_get_time(void) {
unsigned char year, month, day, hour, minute, second;
ds1302_write_byte(0xbf); // 写入开始读取时间的命令字节
second = ds1302_read_byte() & 0x7f; // 读取秒数,将最高位清零以避免闰秒的影响
minute = ds1302_read_byte(); // 读取分钟
hour = ds1302_read_byte(); // 读取小时
day = ds1302_read_byte(); // 读取日期
month = ds1302_read_byte(); // 读取月份
year = ds1302_read_byte(); // 读取年份
ds1302_read_byte(); // 读取星期几,此处不使用
ds1302_read_byte(); // 读取保留字节,此处不使用
ds1302_set_time(year, month, day, hour, minute, second); // 将读取到的时间设置回DS1302芯片中
}
unsigned char ds1302_get_seconds(void) {
return ds1302_read_byte() & 0x7f; // 读取秒数,将最高位清零以避免闰秒的影响
}
unsigned char ds1302_get_minutes(void) {
return ds1302_read_byte(); // 读取分钟
}
unsigned char ds1302_get_hours(void) {
return ds1302_read_byte(); // 读取小时
}
unsigned char ds1302_get_day_of_week(void) {
ds1302_read_byte(); // 读取星期几,此处不使用
return 0;
}
unsigned char ds1302_get_day_of_month(void) {
return ds1302_read_byte(); // 读取日期
}
unsigned char ds1302_get_month(void) {
return ds1302_read_byte(); // 读取月份
}
unsigned char ds1302_get_year(void) {
return ds1302_read_byte(); // 读取年份
}
```
在`main`函数中,我们使用`ds1302_init`函数来初始化DS1302实时时钟芯片,使用`ds1302_get_time`函数来获取当前时间,使用`ds1302_get_seconds`函数来获取当前秒数。如果当前秒数为0,则表示已经进入下一分钟,在此处可以添加闹钟检测代码。
需要注意的是,上述代码中的延时函数`__delay_cycles`的参数是以CPU时钟周期为单位的,因此需要根据实际的CPU时钟频率来调整参数。
相关推荐
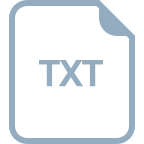
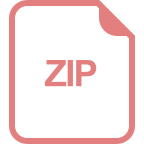














