python画动态星空图代码
时间: 2023-03-29 18:03:47 浏览: 179
以下是 Python 画动态星空图的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
fig, ax = plt.subplots()
# 生成随机的星星坐标
stars = np.random.rand(100, 2)
# 绘制星空图
scatter = ax.scatter(stars[:, ], stars[:, 1], s=5)
# 定义动画函数
def update(frame):
# 每一帧都将星星的位置随机移动一点
stars[:, ] += np.random.randn(100) * .01
stars[:, 1] += np.random.randn(100) * .01
# 限制星星的位置在 [, 1] 范围内
stars[:, ] = np.clip(stars[:, ], , 1)
stars[:, 1] = np.clip(stars[:, 1], , 1)
# 更新星星的位置
scatter.set_offsets(stars)
return scatter,
# 创建动画
ani = animation.FuncAnimation(fig, update, interval=50)
plt.show()
```
希望对你有帮助!
相关问题
python画动态星空图
可以使用 Python 的 matplotlib 库来画动态星空图。下面是一个简单的实现:
```python
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
fig, ax = plt.subplots()
# 生成一些随机的星星
num_stars = 100
stars = np.random.rand(num_stars, 2) * 2 - 1
# 绘制星空图
ax.scatter(stars[:, 0], stars[:, 1], s=1, color='white')
# 设置坐标轴范围和隐藏坐标轴
ax.set_xlim(-1, 1)
ax.set_ylim(-1, 1)
ax.set_axis_off()
# 定义动画函数
def update(frame):
# 每帧都将星星随机移动一小步
step_size = 0.01
stars[:, 0] += np.random.randn(num_stars) * step_size
stars[:, 1] += np.random.randn(num_stars) * step_size
# 将移动后的星星重新绘制到图上
ax.clear()
ax.scatter(stars[:, 0], stars[:, 1], s=1, color='white')
ax.set_xlim(-1, 1)
ax.set_ylim(-1, 1)
ax.set_axis_off()
# 创建动画对象
ani = animation.FuncAnimation(fig, update, frames=100, interval=50)
# 显示动画
plt.show()
```
运行代码后,会弹出一个窗口,显示动态的星空图。每帧都会将星星随机移动一小步,从而产生动画效果。可以根据自己的需要修改代码,例如修改星星的数量、颜色等。
用python画梵高星空的代码
以下是用Python绘制梵高星空的代码:
```python
import turtle
import random
# 设置画布大小和背景颜色
turtle.setup(800, 600)
turtle.bgcolor("#000000")
# 定义星星的颜色和大小
colors = ["#FFFFFF", "#FFFF00", "#FF00FF", "#00FFFF"]
sizes = [1, 2, 3]
# 定义绘制星星的函数
def draw_star(x, y):
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.color(random.choice(colors))
turtle.begin_fill()
size = random.choice(sizes)
for i in range(5):
turtle.forward(size * 10)
turtle.right(144)
turtle.end_fill()
# 绘制星空
for i in range(200):
x = random.randint(-400, 400)
y = random.randint(-300, 300)
draw_star(x, y)
# 隐藏画笔
turtle.hideturtle()
# 显示绘图窗口
turtle.done()
```
阅读全文
相关推荐
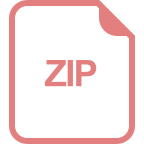
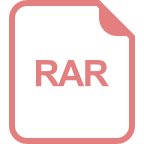
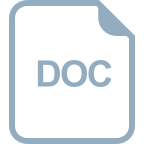



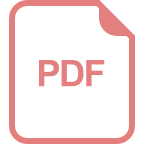
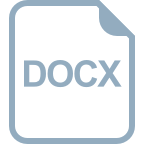
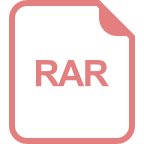
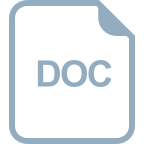
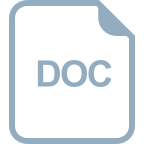
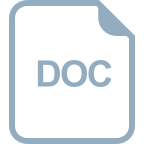



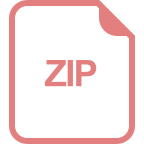
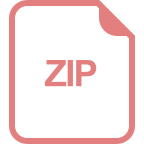