python 使用拉普拉斯锐化图像
时间: 2023-10-24 22:11:02 浏览: 140
拉普拉斯锐化是一种图像增强的技术,可以使图像更加清晰。在Python中,可以使用OpenCV库实现图像的拉普拉斯锐化。下面是一个简单的代码示例:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.jpg')
# 定义拉普拉斯核
kernel = np.array([[-1,-1,-1],[-1,9,-1],[-1,-1,-1]])
# 进行拉普拉斯锐化
sharp_img = cv2.filter2D(img, -1, kernel)
# 显示原始图像和锐化后的图像
cv2.imshow('Original Image', img)
cv2.imshow('Sharpened Image', sharp_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在代码中,首先读取了一张图像,然后定义了一个3x3的拉普拉斯核。接着使用`cv2.filter2D()`函数对图像进行拉普拉斯锐化,最后显示原始图像和锐化后的图像。你可以根据需要修改代码中的图像路径和拉普拉斯核的大小。
相关问题
python图像拉普拉斯锐化
图像拉普拉斯锐化可以通过以下步骤实现:
1. 导入必要的库和图像
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
```
2. 定义拉普拉斯核
```python
laplacian_kernel = np.array([[0, 1, 0], [1, -4, 1], [0, 1, 0]])
```
3. 对图像进行卷积操作
```python
laplacian_img = cv2.filter2D(img, -1, laplacian_kernel)
```
4. 将卷积结果与原图像相加,得到锐化后的图像
```python
sharpened_img = cv2.addWeighted(img, 1.5, laplacian_img, -0.5, 0)
```
完整代码如下:
```python
import cv2
import numpy as np
img = cv2.imread('image.jpg')
laplacian_kernel = np.array([[0, 1, 0], [1, -4, 1], [0, 1, 0]])
laplacian_img = cv2.filter2D(img, -1, laplacian_kernel)
sharpened_img = cv2.addWeighted(img, 1.5, laplacian_img, -0.5, 0)
cv2.imshow('Original', img)
cv2.imshow('Sharpened', sharpened_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
运行代码后,可以看到原图像和锐化后的图像。
python实现拉普拉斯锐化(不使用cv2库),
以下是一种实现方式:
```python
import numpy as np
import matplotlib.pyplot as plt
from PIL import Image
def laplace_sharpen(image):
# 定义拉普拉斯卷积核
laplace_kernel = np.array([[0, -1, 0], [-1, 4, -1], [0, -1, 0]])
# 将图像转换为灰度图像
gray_image = image.convert('L')
# 转换为numpy数组
image_array = np.array(gray_image)
# 对图像进行卷积操作
sharpened_image_array = np.zeros(image_array.shape)
for i in range(1, image_array.shape[0] - 1):
for j in range(1, image_array.shape[1] - 1):
sharpened_image_array[i, j] = np.sum(laplace_kernel * image_array[i-1:i+2, j-1:j+2])
# 将像素值缩放到0-255之间
sharpened_image_array = (sharpened_image_array - np.min(sharpened_image_array)) / (np.max(sharpened_image_array) - np.min(sharpened_image_array)) * 255
# 将数组转换为图像
sharpened_image = Image.fromarray(sharpened_image_array.astype(np.uint8))
return sharpened_image
# 读取图像
image = Image.open('test.jpg')
# 进行拉普拉斯锐化
sharpened_image = laplace_sharpen(image)
# 显示原图和处理后的图像
fig, ax = plt.subplots(1, 2, figsize=(8, 4))
ax[0].imshow(image)
ax[0].set_title('Original Image')
ax[1].imshow(sharpened_image)
ax[1].set_title('Sharpened Image')
plt.show()
```
需要注意的是,这种实现方式只适用于灰度图像,如果要对彩色图像进行拉普拉斯锐化,需要将每个通道分别进行处理。另外,这种实现方式可能会导致输出图像的像素值超出0-255的范围,需要进行像素值缩放操作。
阅读全文
相关推荐
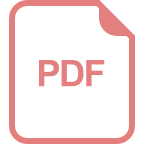













