基于VCGlib的流形修复各种算法及对应示例
时间: 2023-09-11 18:06:59 浏览: 156
基于VCGlib的流形修复算法可以分为以下几种:
1. Poisson Surface Reconstruction
Poisson Surface Reconstruction是一种基于点云的流形重建算法,它通过对点云进行Poisson方程的求解,得到一个连续的曲面模型。该算法的优点是能够处理具有复杂拓扑结构的点云,并且所得到的曲面模型具有高质量的光滑性和细节保留能力。
示例代码:
```c++
#include <vcg/complex/algorithms/create/platonic.h>
#include <vcg/complex/algorithms/update/topology.h>
#include <vcg/complex/algorithms/update/bounding.h>
#include <vcg/complex/algorithms/clean.h>
#include <vcg/complex/algorithms/poisson.h>
using namespace vcg;
int main()
{
// 创建一个四面体网格模型
CMeshO mesh;
tet::Tetrahedron(mesh);
// 对模型进行拓扑更新和边界计算
UpdateTopology<CMeshO>::FaceFace(mesh);
UpdateBounding<CMeshO>::Box(mesh);
// 清除无效面和顶点
tri::Clean<CMeshO>::RemoveDuplicateVertex(mesh);
tri::Clean<CMeshO>::RemoveUnreferencedVertex(mesh);
tri::Clean<CMeshO>::RemoveSmallConnectedComponentsSize<CMeshO>(mesh, 1);
// 进行流形重建
tri::Poisson<CMeshO>::ComputeMesh(mesh);
// 输出结果
tri::io::ExporterOBJ<CMeshO>::Save(mesh, "output.obj", tri::io::Mask::IOM_VERTCOORD);
return 0;
}
```
2. Screened Poisson Surface Reconstruction
Screened Poisson Surface Reconstruction是一种基于点云的流形重建算法,它与Poisson Surface Reconstruction非常相似,但是在求解Poisson方程时,引入了一个约束矩阵,从而能够更好地处理噪声点和边界点。
示例代码:
```c++
#include <vcg/complex/algorithms/create/platonic.h>
#include <vcg/complex/algorithms/update/topology.h>
#include <vcg/complex/algorithms/update/bounding.h>
#include <vcg/complex/algorithms/clean.h>
#include <vcg/complex/algorithms/screened_poisson.h>
using namespace vcg;
int main()
{
// 创建一个四面体网格模型
CMeshO mesh;
tet::Tetrahedron(mesh);
// 对模型进行拓扑更新和边界计算
UpdateTopology<CMeshO>::FaceFace(mesh);
UpdateBounding<CMeshO>::Box(mesh);
// 清除无效面和顶点
tri::Clean<CMeshO>::RemoveDuplicateVertex(mesh);
tri::Clean<CMeshO>::RemoveUnreferencedVertex(mesh);
tri::Clean<CMeshO>::RemoveSmallConnectedComponentsSize<CMeshO>(mesh, 1);
// 进行流形重建
tri::ScreenedPoisson<CMeshO>::ComputeMesh(mesh);
// 输出结果
tri::io::ExporterOBJ<CMeshO>::Save(mesh, "output.obj", tri::io::Mask::IOM_VERTCOORD);
return 0;
}
```
3. Ball-Pivoting Algorithm
Ball-Pivoting Algorithm是一种基于三角网格的流形修复算法,它通过在三角网格上进行球面轮廓扫描,并从中选择合适的边来构建新的三角形,最终得到一个连续的曲面模型。该算法的优点是能够处理具有大规模点云的情况,并且所得到的曲面模型具有较好的几何和拓扑性质。
示例代码:
```c++
#include <vcg/complex/algorithms/create/platonic.h>
#include <vcg/complex/algorithms/update/topology.h>
#include <vcg/complex/algorithms/update/bounding.h>
#include <vcg/complex/algorithms/clean.h>
#include <vcg/complex/algorithms/ball_pivoting.h>
using namespace vcg;
int main()
{
// 创建一个四面体网格模型
CMeshO mesh;
tet::Tetrahedron(mesh);
// 对模型进行拓扑更新和边界计算
UpdateTopology<CMeshO>::FaceFace(mesh);
UpdateBounding<CMeshO>::Box(mesh);
// 清除无效面和顶点
tri::Clean<CMeshO>::RemoveDuplicateVertex(mesh);
tri::Clean<CMeshO>::RemoveUnreferencedVertex(mesh);
tri::Clean<CMeshO>::RemoveSmallConnectedComponentsSize<CMeshO>(mesh, 1);
// 进行流形重建
tri::BallPivoting<CMeshO>::ComputeMesh(mesh);
// 输出结果
tri::io::ExporterOBJ<CMeshO>::Save(mesh, "output.obj", tri::io::Mask::IOM_VERTCOORD);
return 0;
}
```
以上是基于VCGlib的流形修复算法及对应示例,希望能对你有所帮助。
阅读全文
相关推荐







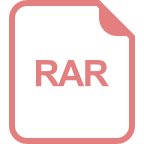





