c++ 手写map链表类
时间: 2023-09-06 12:11:55 浏览: 119
以下是一个简单的基于链表实现的手写Map类示例,你可以参考一下:
```c++
#include <iostream>
#include <string>
using namespace std;
template <typename KeyType, typename ValueType>
class Map {
private:
struct Node {
KeyType key;
ValueType value;
Node* next;
Node(const KeyType& k, const ValueType& v) : key(k), value(v), next(nullptr) {}
};
Node* head;
public:
Map() : head(nullptr) {}
~Map() {
Node* node = head;
while (node != nullptr) {
Node* next = node->next;
delete node;
node = next;
}
}
void insert(const KeyType& k, const ValueType& v) {
Node* node = head;
while (node != nullptr) {
if (node->key == k) {
node->value = v;
return;
}
node = node->next;
}
node = new Node(k, v);
node->next = head;
head = node;
}
const ValueType& operator[](const KeyType& k) const {
Node* node = head;
while (node != nullptr) {
if (node->key == k) {
return node->value;
}
node = node->next;
}
throw "Key not found!";
}
bool contains(const KeyType& k) const {
Node* node = head;
while (node != nullptr) {
if (node->key == k) {
return true;
}
node = node->next;
}
return false;
}
};
int main() {
Map<string, int> myMap;
myMap.insert("apple", 3);
myMap.insert("banana", 6);
myMap.insert("cherry", 9);
cout << myMap["apple"] << endl; // 3
cout << myMap["banana"] << endl; // 6
cout << myMap["cherry"] << endl; // 9
cout << myMap["durian"] << endl; // throws "Key not found!"
if (myMap.contains("apple")) {
cout << "myMap contains 'apple'" << endl;
}
if (!myMap.contains("durian")) {
cout << "myMap does not contain 'durian'" << endl;
}
return 0;
}
```
在这个示例中,我们使用了一个简单的单向链表来存储键值对。Map类提供了三个基本的操作:insert、operator[]和contains。insert操作将一个键值对插入到链表中,如果键已经存在,则更新对应的值。operator[]操作用于获取特定键的值,如果键不存在,则抛出异常。contains操作用于检查特定键是否存在于Map中。
阅读全文
相关推荐



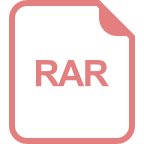



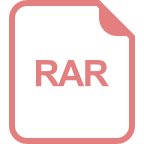
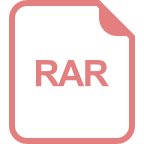
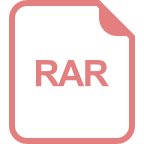

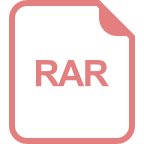






